sched_int 中没有找到init_waitqueue_head
时间: 2023-08-05 08:06:07 浏览: 53
非常抱歉,我的回答有误。在 Linux 内核中,进程调度的等待队列是在 `sched_fork()` 函数中初始化的,而不是在 `sched_init()` 函数中。
具体地说,`sched_fork()` 函数是在进程创建(fork)时被调用的,它负责初始化新创建的进程的调度信息。在该函数中,会调用 `__init_waitqueue_head(&p->wait_chldexit)` 函数,初始化名为 `wait_chldexit` 的等待队列头部,用于等待子进程退出的信号。
`__init_waitqueue_head()` 函数是一个内核中的帮助函数,它的作用是初始化一个等待队列头部节点。具体来说,该函数使用 `__WAIT_QUEUE_HEAD_INITIALIZER()` 宏将等待队列头部节点初始化为一个空的节点,然后将其赋值给等待队列头部变量,完成等待队列的初始化。
需要注意的是,进程调度的等待队列头部变量名为 `wait_chldexit`,它是一个进程结构体中的成员变量,每个进程都有自己的等待队列头部。在实际编程中,为了避免竞争条件等问题,需要使用相关的同步机制来保证进程访问等待队列的正确性。
相关推荐
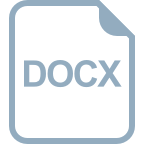
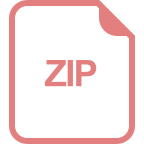
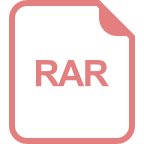
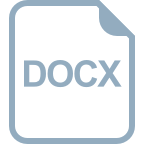
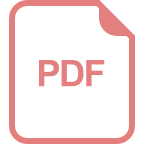
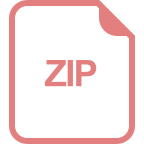
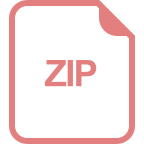
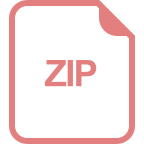
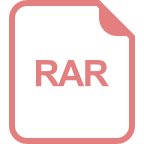