springboot中bean的注入过程
时间: 2024-05-31 19:15:05 浏览: 168
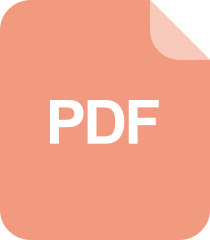
Springboot测试类没有bean注入问题解析

在 Spring Boot 中,Bean 的注入过程大致如下:
1. 扫描项目中的注解
Spring Boot 会自动扫描项目中所有被 `@Component` 及其派生注解(如 `@Service`、`@Repository` 等)所标注的类,将这些类注册到 Spring 的 Bean 容器中。
2. 注入属性
Spring Boot 会检查被 `@Autowired` 注解标记的属性,并尝试为其注入对应的 Bean。如果存在多个符合条件的 Bean,则根据字段类型、字段名称和 `@Qualifier` 注解进行匹配。
3. 创建 Bean
如果 Spring Boot 扫描到一个类被 `@Configuration` 注解标记,那么 Spring Boot 会创建一个 Bean 配置类,并将其中标记了 `@Bean` 注解的方法所返回的对象注册到 Spring 的 Bean 容器中。
4. 处理依赖关系
在 Bean 注入过程中,Spring Boot 会检查 Bean 之间的依赖关系,并按照依赖关系的顺序进行实例化和注入。
5. 初始化 Bean
在 Bean 实例化和属性注入完成之后,Spring Boot 会调用 `@PostConstruct` 注解所标注的方法进行 Bean 的初始化。
6. 销毁 Bean
在 Spring Boot 应用关闭时,Spring 会调用 `@PreDestroy` 注解所标注的方法来销毁 Bean。
阅读全文
相关推荐
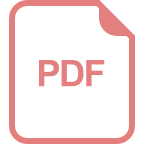











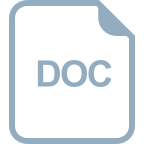



