怎么用opencv进行数字识别
时间: 2023-08-17 07:50:54 浏览: 181
使用OpenCV进行数字识别的一种常见方法是利用图像处理和机器学习技术。下面是一个简单的步骤指南:
1. 导入所需的库和模块:
```python
import cv2
import numpy as np
from sklearn.externals import joblib
```
2. 加载训练好的数字识别模型:
```python
model = joblib.load('digit_model.pkl')
```
这里的 `digit_model.pkl` 是已经训练好的模型文件。
3. 读取待识别的图像并进行预处理:
```python
image = cv2.imread('input_image.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
thresh = cv2.adaptiveThreshold(blurred, 255, cv2.ADAPTIVE_THRESH_GAUSSIAN_C, cv2.THRESH_BINARY_INV, 11, 2)
```
这里的 `input_image.jpg` 是待识别的图像文件。
4. 检测图像中的数字轮廓:
```python
contours, hierarchy = cv2.findContours(thresh.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
```
5. 对每个数字轮廓进行识别:
```python
for contour in contours:
(x, y, w, h) = cv2.boundingRect(contour)
if w >= 5 and h >= 25:
roi = thresh[y:y + h, x:x + w]
roi = np.pad(roi, (5, 5), 'constant', constant_values=0)
roi = cv2.resize(roi, (28, 28))
roi = np.array(roi, dtype='float32').reshape(1, 784)
roi /= 255
digit = model.predict(roi)
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.putText(image, str(digit), (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 255, 0), 2)
```
6. 显示识别结果:
```python
cv2.imshow("Result", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
以上是用OpenCV进行数字识别的一个简单示例。你可以根据自己的需求和数据集进行进一步的调整和优化。
阅读全文
相关推荐
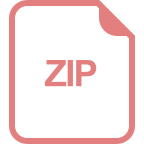
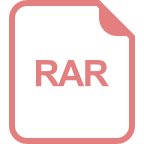
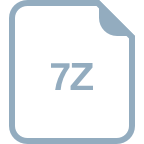







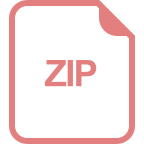
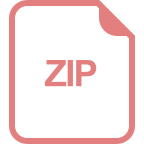
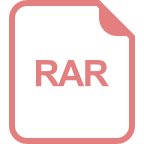
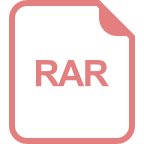
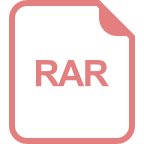
