java断点续传前后端交互实现代码
时间: 2023-06-29 09:10:43 浏览: 91
Java断点续传需要前后端交互实现,以下是一个简单的示例代码:
前端代码(使用jQuery):
```javascript
$(function() {
var fileInput = $('#fileInput');
var fileSize = fileInput[0].files[0].size;
var startByte = 0;
$('#uploadButton').click(function() {
var formData = new FormData();
formData.append('file', fileInput[0].files[0]);
formData.append('startByte', startByte);
$.ajax({
url: '/upload',
type: 'POST',
data: formData,
processData: false,
contentType: false,
xhr: function() {
var xhr = $.ajaxSettings.xhr();
xhr.upload.onprogress = function(e) {
if (e.lengthComputable) {
var percent = Math.round((e.loaded + startByte) / fileSize * 100);
$('#progressBar').css('width', percent + '%');
}
};
return xhr;
},
success: function(response) {
if (response.status === 'success') {
startByte = response.startByte;
if (startByte === fileSize) {
alert('上传完成');
}
} else {
alert('上传失败');
}
},
error: function() {
alert('上传失败');
}
});
});
});
```
后端代码(使用Spring Boot):
```java
@RestController
public class UploadController {
private static final String UPLOAD_DIR = "/path/to/upload/dir";
private static final int BUFFER_SIZE = 1024 * 1024;
@PostMapping("/upload")
public Map<String, Object> upload(@RequestParam("file") MultipartFile file,
@RequestParam("startByte") long startByte) throws IOException {
File uploadDir = new File(UPLOAD_DIR);
if (!uploadDir.exists()) {
uploadDir.mkdirs();
}
File uploadFile = new File(uploadDir, file.getOriginalFilename());
RandomAccessFile raf = new RandomAccessFile(uploadFile, "rw");
raf.seek(startByte);
InputStream in = file.getInputStream();
byte[] buffer = new byte[BUFFER_SIZE];
int len = 0;
long totalBytesRead = startByte;
while ((len = in.read(buffer)) != -1) {
raf.write(buffer, 0, len);
totalBytesRead += len;
}
raf.close();
in.close();
Map<String, Object> response = new HashMap<>();
response.put("status", "success");
response.put("startByte", totalBytesRead);
return response;
}
}
```
以上代码仅供参考,实际使用时需要根据具体需求进行修改。
阅读全文
相关推荐


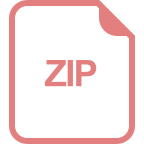
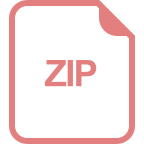
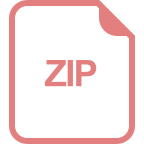
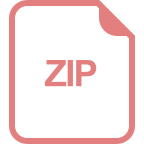
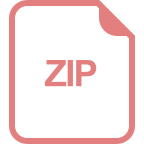
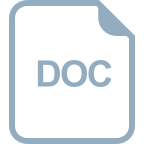
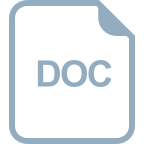
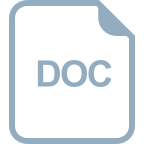
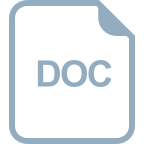
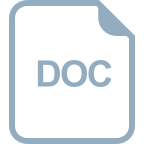
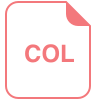
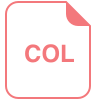
