用Java定义一个有理数类,实现有理数的基本运算:加、减、乘、除,约分。
时间: 2023-06-02 13:06:58 浏览: 51
好的,这是一个关于有理数类的编程问题。以下是用Java定义一个有理数类的基本代码:
```
public class RationalNumber {
private int numerator; // 分子
private int denominator; // 分母
public RationalNumber(int numerator, int denominator) {
if (denominator == 0) {
throw new IllegalArgumentException("分母不能为0");
}
// 化简分数
int gcd = gcd(numerator, denominator);
this.numerator = numerator / gcd;
this.denominator = denominator / gcd;
// 如果分母为负数,则将负号调整到分子上
if (this.denominator < 0) {
this.numerator = -this.numerator;
this.denominator = -this.denominator;
}
}
// 获取分子
public int getNumerator() {
return numerator;
}
// 获取分母
public int getDenominator() {
return denominator;
}
// 加法
public RationalNumber add(RationalNumber other) {
int top = this.numerator * other.denominator + this.denominator * other.numerator;
int bottom = this.denominator * other.denominator;
return new RationalNumber(top, bottom);
}
// 减法
public RationalNumber subtract(RationalNumber other) {
int top = this.numerator * other.denominator - this.denominator * other.numerator;
int bottom = this.denominator * other.denominator;
return new RationalNumber(top, bottom);
}
// 乘法
public RationalNumber multiply(RationalNumber other) {
int top = this.numerator * other.numerator;
int bottom = this.denominator * other.denominator;
return new RationalNumber(top, bottom);
}
// 除法
public RationalNumber divide(RationalNumber other) {
int top = this.numerator * other.denominator;
int bottom = this.denominator * other.numerator;
return new RationalNumber(top, bottom);
}
// 约分
private int gcd(int a, int b) {
if (b == 0) {
return a;
} else {
return gcd(b, a % b);
}
}
// 转化为字符串形式
public String toString() {
if (this.denominator == 1) {
return String.valueOf(this.numerator);
} else {
return this.numerator + "/" + this.denominator;
}
}
}
```
您可以根据自己的需要对这个代码进行修改和补充。希望能对您有所帮助。
相关推荐
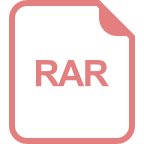














