C++随机生成一个5*5的二维数组
时间: 2023-12-31 07:04:43 浏览: 42
下面是使用C++标准库的随机数生成器生成一个5*5的二维数组的示例代码:
```c++
#include <iostream>
#include <random>
const int ROWS = 5;
const int COLS = 5;
int main()
{
std::random_device rd;
std::mt19937 gen(rd());
std::uniform_int_distribution<> distr(1, 100);
int arr[ROWS][COLS];
for (int i = 0; i < ROWS; i++)
{
for (int j = 0; j < COLS; j++)
{
arr[i][j] = distr(gen);
std::cout << arr[i][j] << " ";
}
std::cout << std::endl;
}
return 0;
}
```
这个程序使用了C++11标准库中的随机数生成器`std::mt19937`和均匀分布器`std::uniform_int_distribution`。在每个数组元素中生成一个1到100之间的随机整数。
相关问题
c++随机生成二维数组
以下是使用C++随机生成二维数组的示例代码:
```c++
#include <iostream>
#include <stdlib.h>
#include <time.h>
using namespace std;
int** generateRandomArray(int n, int m) {
int** arr = new int*[n];
srand((unsigned)time(NULL));
for (int i = 0; i < n; i++) {
arr[i] = new int[m];
for (int j = 0; j < m; j++) {
arr[i][j] = (rand() % 900) + 100;
}
}
return arr;
}
void printArray(int** arr, int n, int m) {
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
cout << arr[i][j] << " ";
}
cout << endl;
}
}
int main() {
int n, m;
cin >> n >> m;
int** arr = generateRandomArray(n, m);
printArray(arr, n, m);
return 0;
}
```
C++生成随机二维数组
以下是使用C++生成随机二维数组的示例:
```cpp
#include <iostream>
#include <stdlib.h>
#include <time.h>
using namespace std;
int main(){
int n, m;
cin >> n >> m;
srand((unsigned)time(NULL));
for(int i = 0; i < n; i++){
for(int j = 0; j < m; j++){
cout << (rand() % 900) + 100 << " ";
}
cout << endl;
}
return 0;
}
```
相关推荐
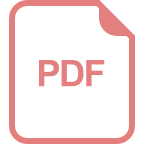
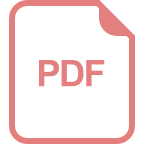
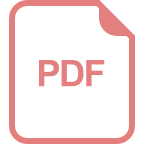













