python读取excel的数据并绘制词云图
时间: 2023-08-11 08:41:28 浏览: 34
好的,您可以按照以下步骤来实现:
1. 首先,需要安装一些需要的库,比如 pandas、jieba、wordcloud。您可以使用以下命令来安装它们:
```
pip install pandas jieba wordcloud
```
2. 接着,导入需要的库:
```python
import pandas as pd
import jieba
from wordcloud import WordCloud
import matplotlib.pyplot as plt
```
3. 读取 Excel 文件中的数据。假设您的 Excel 文件名为 data.xlsx,里面的数据存储在名为 sheet1 的工作表中,数据列名为 content,那么您可以使用以下代码来读取数据:
```python
df = pd.read_excel('data.xlsx', sheet_name='sheet1')
data = df['content'].tolist()
```
4. 对文本进行分词处理。使用 jieba 库,您可以使用以下代码来对文本进行分词:
```python
stopwords = [line.strip() for line in open('stopwords.txt', 'r', encoding='utf-8').readlines()]
words = []
for text in data:
seg_list = jieba.cut(text, cut_all=False)
for word in seg_list:
if word not in stopwords:
words.append(word)
```
其中 stopwords.txt 是存储停用词的文件,您需要提前下载并存储在项目目录中。
5. 绘制词云图。使用 WordCloud 库,您可以使用以下代码来绘制词云图:
```python
text = ' '.join(words)
wordcloud = WordCloud(background_color="white", width=800, height=600).generate(text)
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis("off")
plt.show()
```
这里使用了 matplotlib 库来显示词云图。
完整代码如下:
```python
import pandas as pd
import jieba
from wordcloud import WordCloud
import matplotlib.pyplot as plt
df = pd.read_excel('data.xlsx', sheet_name='sheet1')
data = df['content'].tolist()
stopwords = [line.strip() for line in open('stopwords.txt', 'r', encoding='utf-8').readlines()]
words = []
for text in data:
seg_list = jieba.cut(text, cut_all=False)
for word in seg_list:
if word not in stopwords:
words.append(word)
text = ' '.join(words)
wordcloud = WordCloud(background_color="white", width=800, height=600).generate(text)
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis("off")
plt.show()
```
希望这个回答能够帮到您。
相关推荐
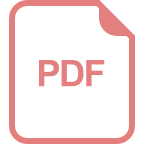
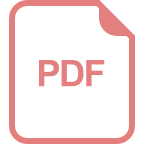
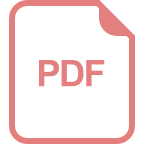
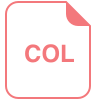
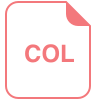
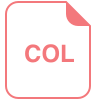
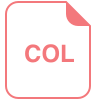
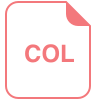









