用c语言写出3. 综合题:有 4 个线程 P1、P2、P3 和 P4 共享一个缓冲区,线程 P1 向缓冲区中存入消息,线程 P2、P3 和 P4 从缓冲区中取消息,要求发送者必须等 3 个进程都取过本条消息后才能发送下一条消息。缓冲区内每次只能容纳 1 个消息,请用 P、V操作描述 4 个进程存取消息的情况,并用代码实现上述过程。
时间: 2023-06-15 11:03:48 浏览: 152
以下是用C语言实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#define BUFFER_SIZE 1
sem_t mutex, empty, full;
int buffer[BUFFER_SIZE];
int in = 0, out = 0;
void *producer(void *);
void *consumer(void *);
int main()
{
pthread_t t1, t2, t3, t4;
sem_init(&mutex, 0, 1);
sem_init(&empty, 0, BUFFER_SIZE);
sem_init(&full, 0, 0);
pthread_create(&t1, NULL, producer, NULL);
pthread_create(&t2, NULL, consumer, NULL);
pthread_create(&t3, NULL, consumer, NULL);
pthread_create(&t4, NULL, consumer, NULL);
pthread_join(t1, NULL);
pthread_join(t2, NULL);
pthread_join(t3, NULL);
pthread_join(t4, NULL);
sem_destroy(&mutex);
sem_destroy(&empty);
sem_destroy(&full);
return 0;
}
void *producer(void *arg)
{
int i, item;
for (i = 0; i < 10; i++)
{
item = rand() % 100 + 1;
sem_wait(&empty);
sem_wait(&mutex);
buffer[in] = item;
printf("Producer produces item %d\n", item);
in = (in + 1) % BUFFER_SIZE;
sem_post(&mutex);
sem_post(&full);
}
pthread_exit(NULL);
}
void *consumer(void *arg)
{
int i, item;
for (i = 0; i < 10; i++)
{
sem_wait(&full);
sem_wait(&mutex);
item = buffer[out];
printf("Consumer %d consumes item %d\n", (int)arg, item);
out = (out + 1) % BUFFER_SIZE;
if (out == 0)
{
sem_post(&empty);
}
sem_post(&mutex);
}
pthread_exit(NULL);
}
```
在上述代码中,我们使用了三个信号量来实现互斥、同步和缓冲区空间的控制:`mutex` 用于保证生产者和消费者的互斥访问;`empty` 用于控制缓冲区空间的使用;`full` 用于控制缓冲区中数据的数量。
在 `producer` 函数中,生产者会随机生成一个整数,并尝试将其存入缓冲区中。在存入数据之前,生产者需要先等待 `empty` 信号量,保证缓冲区中有空闲空间;然后再等待 `mutex` 信号量,保证生产者和消费者之间的互斥访问;最后再将数据存入缓冲区,并更新 `in` 指针,最后释放 `mutex` 和 `full` 信号量,表示数据已经可供消费者使用。
在 `consumer` 函数中,消费者需要从缓冲区中取出数据,如果缓冲区为空,则等待 `full` 信号量。然后再等待 `mutex` 信号量,保证生产者和消费者之间的互斥访问;然后将数据从缓冲区中取出,并更新 `out` 指针;如果缓冲区已满,则释放 `empty` 信号量,表示缓冲区中有空闲空间;最后释放 `mutex` 信号量,表示数据已经被消费者使用完毕。
通过以上的代码实现,我们可以保证生产者和消费者之间的同步,同时保证缓冲区中的数据量不会超过其容量,从而实现了多线程程序的正确性。
阅读全文
相关推荐
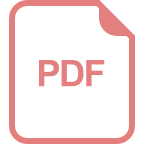
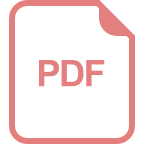
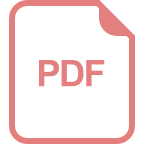
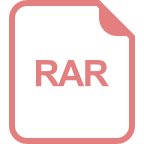
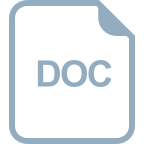
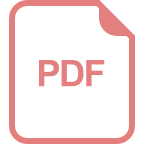
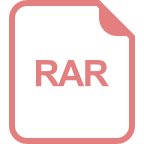
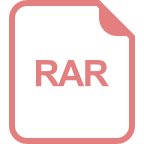
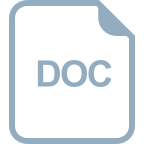
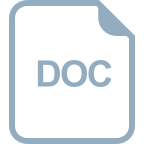
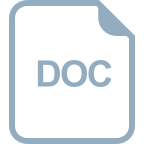
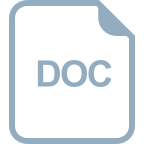
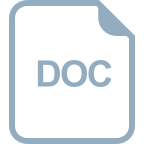
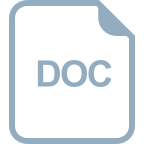
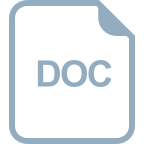
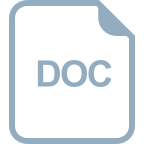