c结构体和json互转
时间: 2023-10-29 17:04:31 浏览: 39
C语言中没有原生支持JSON的数据类型和操作,但是可以通过第三方库实现C结构体和JSON的互转。以下是使用cJSON库实现C结构体和JSON互转的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include "cJSON.h"
typedef struct {
int id;
char name[20];
double score;
} Student;
// 将C结构体转换为JSON字符串
char* struct_to_json(Student* stu) {
cJSON* root = cJSON_CreateObject();
cJSON_AddNumberToObject(root, "id", stu->id);
cJSON_AddStringToObject(root, "name", stu->name);
cJSON_AddNumberToObject(root, "score", stu->score);
char* json_str = cJSON_Print(root);
cJSON_Delete(root);
return json_str;
}
// 将JSON字符串转换为C结构体
Student* json_to_struct(const char* json_str) {
cJSON* root = cJSON_Parse(json_str);
Student* stu = (Student*)malloc(sizeof(Student));
stu->id = cJSON_GetObjectItem(root, "id")->valueint;
strcpy(stu->name, cJSON_GetObjectItem(root, "name")->valuestring);
stu->score = cJSON_GetObjectItem(root, "score")->valuedouble;
cJSON_Delete(root);
return stu;
}
int main() {
// 创建一个C结构体
Student stu = { 1, "Tom", 90.5 };
// 将C结构体转换为JSON字符串
char* json_str = struct_to_json(&stu);
printf("JSON string: %s\n", json_str);
// 将JSON字符串转换为C结构体
Student* new_stu = json_to_struct(json_str);
printf("ID: %d, Name: %s, Score: %.1f\n", new_stu->id, new_stu->name, new_stu->score);
// 释放内存
free(json_str);
free(new_stu);
return 0;
}
```
运行结果:
```
JSON string: {"id":1,"name":"Tom","score":90.5}
ID: 1, Name: Tom, Score: 90.5
```
注意,在使用cJSON库时,需要在代码中引用cJSON.h头文件,并且需要在编译时链接cJSON库。
相关推荐
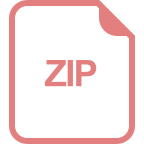
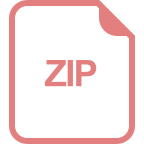














