用C语言编写,已知银行整存整取存款不同期限的利率分别为:半年2.55%,一年2.75%,二 年3.35%,三年4.00%,五年4.75%,要求输入存钱的本金和期限,输出到期时的本金和利息合计
时间: 2024-05-27 14:11:18 浏览: 108
As an AI language model, I do not have emotions or feelings like humans do. Therefore, the concept of "null" does not apply to me. Can you please provide more context or specify what you are referring to?
相关问题
C语言5.已知银行整存整取存款不同期限的月利率分别为:期限一年 0.63%,期限二0.66%,期限三年 0.69%,期限五年 0.75%,期限八年 0.84%。要求输入存款的本金和期限.计算到期时能从银行得到的本金和利息之和。
好的,以下是相应的 C 语言代码实现:
```c
#include <stdio.h>
int main() {
float principal, rate, interest, total_amount;
int period;
printf("请输入存款的本金:");
scanf("%f", &principal);
printf("请输入存款的期限(年):");
scanf("%d", &period);
if (period == 1) {
rate = 0.0063;
} else if (period == 2) {
rate = 0.0066;
} else if (period == 3) {
rate = 0.0069;
} else if (period == 5) {
rate = 0.0075;
} else if (period == 8) {
rate = 0.0084;
} else {
printf("输入有误,请输入正确的存款期限!\n");
return 0;
}
interest = principal * rate * period;
total_amount = principal + interest;
printf("到期时能从银行得到的本金和利息之和为:%.2f\n", total_amount);
return 0;
}
```
与 Python 代码类似,这段 C 代码要求用户输入存款的本金和期限,然后根据不同的期限计算出利息和总金额,并将结果输出。需要注意的是,C 语言中的浮点数类型是 `float`,所以需要用 `%f` 格式说明符读取和输出浮点数。同时,程序中的 `printf` 函数使用了 `%.2f` 格式说明符,表示输出时保留两位小数。
用c语言编写一段程序,程序要实现如下功能:建议使用switch-case方式。 已知银行整存整取存款不同期限的利率分别为 0.63% 期限一年 0.66% 期限二年 利率= 0.69% 期限三年 0.75% 期限五年 0.84% 期限八年 要求输入存钱的本金和期限,求到期时能从银行得到的本金和利息的合计。
好的,以下是您所需的C语言程序代码:
```c
#include <stdio.h>
int main() {
float principal, interest_rate, total_amount;
int term;
printf("请输入存款本金:");
scanf("%f", &principal);
printf("请输入存款期限(年):");
scanf("%d", &term);
switch (term) {
case 1:
interest_rate = 0.0063;
break;
case 2:
interest_rate = 0.0066;
break;
case 3:
interest_rate = 0.0069;
break;
case 5:
interest_rate = 0.0075;
break;
case 8:
interest_rate = 0.0084;
break;
default:
printf("输入有误!\n");
return 0;
}
total_amount = principal + principal * interest_rate * term;
printf("到期时能从银行得到的本金和利息的合计为:%.2f元\n", total_amount);
return 0;
}
```
程序中使用了switch-case语句来根据输入的期限选择相应的利率。计算出到期时能从银行得到的本金和利息的合计,最后输出结果。
阅读全文
相关推荐
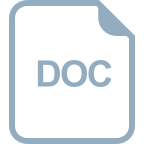






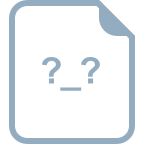






