自定义类型例如struct Student或class Student如何重载<、==、>、<<等运算符;重载目的?
时间: 2023-12-24 16:04:16 浏览: 43
在C++中,我们可以通过重载运算符来改变自定义类型的行为,使其与内置类型一样灵活。例如,我们可以通过重载运算符来比较两个自定义类型对象的大小,或者通过重载运算符来打印一个自定义类型对象的信息。
下面是一个使用class定义的Student类的示例,演示如何重载运算符:
```c++
#include <iostream>
#include <string>
class Student {
private:
std::string name;
int age;
double score;
public:
Student(std::string name, int age, double score) : name(name), age(age), score(score) {}
bool operator<(const Student& other) const {
return score < other.score;
}
bool operator==(const Student& other) const {
return name == other.name && age == other.age && score == other.score;
}
friend std::ostream& operator<<(std::ostream& os, const Student& student) {
os << "Name: " << student.name << ", ";
os << "Age: " << student.age << ", ";
os << "Score: " << student.score;
return os;
}
};
int main() {
Student s1 = {"Alice", 18, 90.5};
Student s2 = {"Bob", 19, 85.5};
Student s3 = {"Charlie", 20, 95.5};
std::cout << (s1 < s2) << std::endl; // 输出 0
std::cout << (s2 < s3) << std::endl; // 输出 1
std::cout << (s1 == s2) << std::endl; // 输出 0
std::cout << s1 << std::endl; // 输出 "Name: Alice, Age: 18, Score: 90.5"
std::cout << s2 << std::endl; // 输出 "Name: Bob, Age: 19, Score: 85.5"
std::cout << s3 << std::endl; // 输出 "Name: Charlie, Age: 20, Score: 95.5"
return 0;
}
```
在上面的示例中,我们定义了一个使用class定义的Student类,并重载了小于运算符、等于运算符和输出运算符。我们可以使用这些运算符来比较两个Student对象的大小,检查它们是否相等,并打印它们的信息。
重载运算符的目的是让自定义类型对象在使用上更加方便,可以像内置类型一样使用运算符来进行操作。同时,重载运算符也可以提高代码的可读性和可维护性。
阅读全文
相关推荐
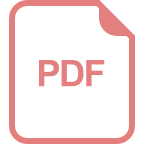
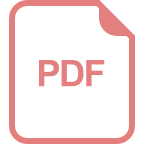




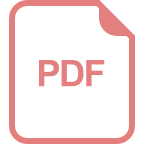
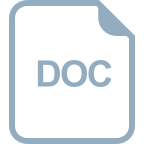
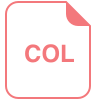
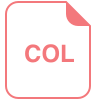
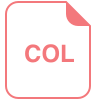
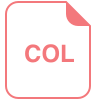




