专科生:学号、姓名、性别、年龄、实习地点 本科生:学号、姓名、性别、年龄、毕业设计题目 研究生:学号、姓名、性别、年龄、指导教师 1.建立类模型、必须抽出基类 2.定义基类(抽象类),对各个属性能进行get和set,将输出函数output()定义为纯虚函数已备在派生类中能输出各数据成员 3.定义派生类,增加相应数据成员,实现设置(set)和得到(get)数据成员,并实现output函数 4.设计本科生类、要求完成构造函数、拷贝构造函数、和析构函数 5.采用友元重载输入''>>"、输出"<<''运算符,完成本科生类的输入输出重载 6.设计一个仿函数对本科生进行比较,规则按照年龄大小(降序)排序,若年龄相同按学号升序排序 7.采用vector和list,编写一个函数完成正向输出所有元素 8.写出主函数,输入若干个本科生放入vector和list容器,调用仿函数完成对本科生的排序,调用7中函数输出所有本科生信息。 用c++实现
时间: 2024-03-24 16:40:58 浏览: 58
基类:
```cpp
class Student {
protected:
int id;
string name;
char gender;
int age;
public:
Student(int id, string name, char gender, int age) : id(id), name(name), gender(gender), age(age) {}
virtual ~Student() {}
int getId() const { return id; }
string getName() const { return name; }
char getGender() const { return gender; }
int getAge() const { return age; }
virtual void output() const = 0;
};
```
本科生类:
```cpp
class Undergraduate : public Student {
private:
string thesisTitle;
public:
Undergraduate(int id, string name, char gender, int age, string thesisTitle) : Student(id, name, gender, age), thesisTitle(thesisTitle) {}
Undergraduate(const Undergraduate& other) : Student(other.id, other.name, other.gender, other.age), thesisTitle(other.thesisTitle) {}
~Undergraduate() {}
string getThesisTitle() const { return thesisTitle; }
void setThesisTitle(const string& thesisTitle) { this->thesisTitle = thesisTitle; }
void output() const {
cout << "Undergraduate student: " << id << ", " << name << ", " << gender << ", " << age << ", " << thesisTitle << endl;
}
friend istream& operator>>(istream& is, Undergraduate& u);
friend ostream& operator<<(ostream& os, const Undergraduate& u);
friend bool operator<(const Undergraduate& u1, const Undergraduate& u2);
};
```
重载输入输出运算符:
```cpp
istream& operator>>(istream& is, Undergraduate& u) {
is >> u.id >> u.name >> u.gender >> u.age >> u.thesisTitle;
return is;
}
ostream& operator<<(ostream& os, const Undergraduate& u) {
os << "Undergraduate student: " << u.id << ", " << u.name << ", " << u.gender << ", " << u.age << ", " << u.thesisTitle << endl;
return os;
}
```
定义仿函数进行排序:
```cpp
struct UndergraduateComparator {
bool operator()(const Undergraduate& u1, const Undergraduate& u2) {
if (u1.getAge() != u2.getAge()) {
return u1.getAge() > u2.getAge(); // 按年龄降序排列
}
return u1.getId() < u2.getId(); // 年龄相同按学号升序排列
}
};
```
使用 vector 和 list 输出所有元素:
```cpp
void outputAll(const vector<Undergraduate>& v, const list<Undergraduate>& l) {
cout << "vector:" << endl;
for (const auto& u : v) {
u.output();
}
cout << "list:" << endl;
for (const auto& u : l) {
u.output();
}
}
```
主函数:
```cpp
int main() {
vector<Undergraduate> v;
list<Undergraduate> l;
int n;
cin >> n;
for (int i = 0; i < n; i++) {
Undergraduate u;
cin >> u;
v.push_back(u);
l.push_back(u);
}
sort(v.begin(), v.end(), UndergraduateComparator());
l.sort(UndergraduateComparator());
outputAll(v, l);
return 0;
}
```
阅读全文
相关推荐
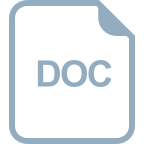
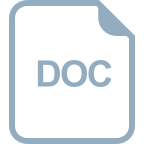
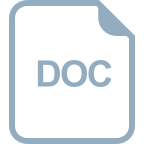



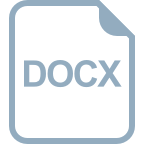
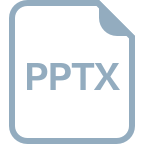
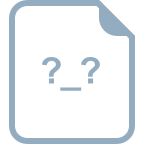
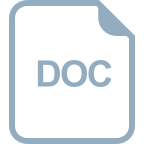
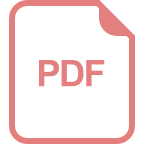
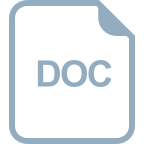
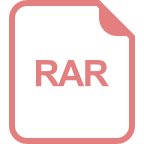
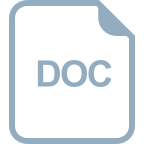
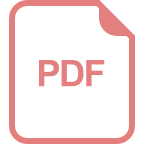
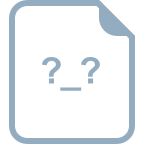
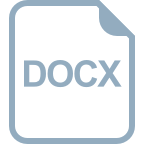
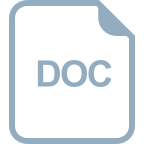
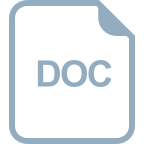