/专科生:学号,姓名,性别,年龄,实习地点 本科生:学号,姓名,性别,年龄,毕业设计题目 研究生:学号,姓名,坐别,年龄,指导教师 定义一个仿函数对本科生进行比较,按照年龄降序排序,若年龄相同按照学号升序排序
时间: 2023-08-11 12:41:50 浏览: 120
可以先定义一个学生基类,包含学号、姓名、性别和年龄等基本信息,然后派生出专科生、本科生和研究生类,分别添加不同的数据成员。最后定义一个仿函数,对本科生进行比较。
具体实现如下:
```c++
#include <iostream>
#include <string>
#include <algorithm>
#include <vector>
class Student {
public:
Student(int id, std::string name, char gender, int age)
: id_(id), name_(std::move(name)), gender_(gender), age_(age) {}
int getId() const { return id_; }
std::string getName() const { return name_; }
char getGender() const { return gender_; }
int getAge() const { return age_; }
bool operator<(const Student& rhs) const {
return age_ == rhs.age_ ? id_ < rhs.id_ : age_ > rhs.age_;
}
private:
int id_;
std::string name_;
char gender_;
int age_;
};
class CollegeStudent : public Student {
public:
CollegeStudent(int id, std::string name, char gender, int age, std::string topic)
: Student(id, std::move(name), gender, age), topic_(std::move(topic)) {}
std::string getTopic() const { return topic_; }
private:
std::string topic_;
};
int main() {
std::vector<CollegeStudent> students{
{1001, "Tom", 'M', 22, "Computer Science"},
{1003, "Jerry", 'M', 20, "Art"},
{1002, "Alice", 'F', 22, "Mathematics"},
};
// 按年龄降序排序,若年龄相同按照学号升序排序
std::sort(students.begin(), students.end());
// 输出结果
for (const auto& student : students) {
std::cout << student.getId() << ", " << student.getName() << ", " << student.getGender()
<< ", " << student.getAge() << ", " << student.getTopic() << std::endl;
}
return 0;
}
```
其中,仿函数重载了小于号运算符,按照题目要求实现了比较逻辑。CollegeStudent类继承自Student类,并添加了毕业设计题目这一数据成员。在main函数中,定义了一个存放CollegeStudent对象的vector容器,对其按照年龄降序排序,若年龄相同按照学号升序排序,然后输出结果。
阅读全文
相关推荐

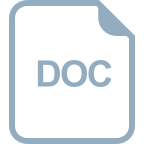
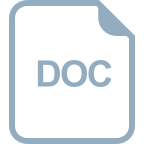








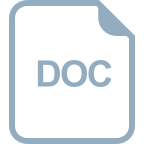

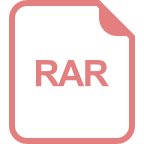

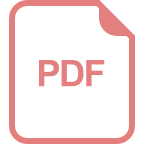


