安卓开发expandablelistview的使用
时间: 2024-02-12 17:09:17 浏览: 123
好的,下面是关于 Android ExpandableListView 的使用方法:
1. 在 layout 文件中添加 ExpandableListView 组件。例如:
```xml
<ExpandableListView
android:id="@+id/my_expandable_listview"
android:layout_width="match_parent"
android:layout_height="match_parent" />
```
2. 创建一个 ExpandableListAdapter 类,继承自 BaseExpandableListAdapter,实现其中的方法。例如:
```java
public class MyExpandableListAdapter extends BaseExpandableListAdapter {
private Context mContext;
private List<String> mGroupData;
private List<List<String>> mChildData;
public MyExpandableListAdapter(Context context, List<String> groupData, List<List<String>> childData) {
mContext = context;
mGroupData = groupData;
mChildData = childData;
}
@Override
public int getGroupCount() {
return mGroupData.size();
}
@Override
public int getChildrenCount(int groupPosition) {
return mChildData.get(groupPosition).size();
}
@Override
public Object getGroup(int groupPosition) {
return mGroupData.get(groupPosition);
}
@Override
public Object getChild(int groupPosition, int childPosition) {
return mChildData.get(groupPosition).get(childPosition);
}
@Override
public long getGroupId(int groupPosition) {
return groupPosition;
}
@Override
public long getChildId(int groupPosition, int childPosition) {
return childPosition;
}
@Override
public boolean hasStableIds() {
return true;
}
@Override
public View getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) {
if (convertView == null) {
convertView = LayoutInflater.from(mContext).inflate(R.layout.group_item_layout, parent, false);
}
TextView groupTextView = convertView.findViewById(R.id.group_textview);
groupTextView.setText(mGroupData.get(groupPosition));
return convertView;
}
@Override
public View getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertView, ViewGroup parent) {
if (convertView == null) {
convertView = LayoutInflater.from(mContext).inflate(R.layout.child_item_layout, parent, false);
}
TextView childTextView = convertView.findViewById(R.id.child_textview);
childTextView.setText(mChildData.get(groupPosition).get(childPosition));
return convertView;
}
@Override
public boolean isChildSelectable(int groupPosition, int childPosition) {
return true;
}
}
```
3. 在 Activity 或 Fragment 中,初始化 ExpandableListView,并设置适配器。例如:
```java
ExpandableListView myExpandableListView = findViewById(R.id.my_expandable_listview);
MyExpandableListAdapter myExpandableListAdapter = new MyExpandableListAdapter(this, groupData, childData);
myExpandableListView.setAdapter(myExpandableListAdapter);
```
4. (可选)设置 ExpandableListView 的监听器,例如监听组展开/收起事件:
```java
myExpandableListView.setOnGroupExpandListener(new ExpandableListView.OnGroupExpandListener() {
@Override
public void onGroupExpand(int groupPosition) {
// 当组展开时的操作
}
});
myExpandableListView.setOnGroupCollapseListener(new ExpandableListView.OnGroupCollapseListener() {
@Override
public void onGroupCollapse(int groupPosition) {
// 当组收起时的操作
}
});
```
这样就完成了 ExpandableListView 的基本使用。需要注意的是,ExpandableListView 中的组和子项都需要自己定义布局,例如在上面的适配器中,分别使用了 group_item_layout 和 child_item_layout 两个布局。
阅读全文
相关推荐
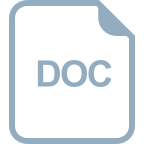
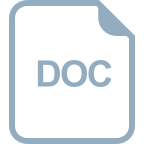
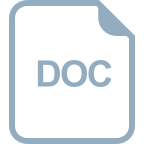

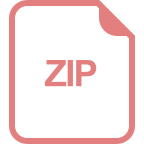
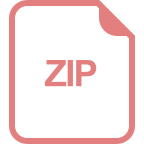
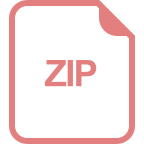
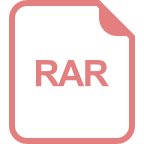
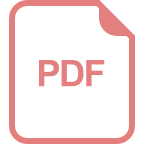
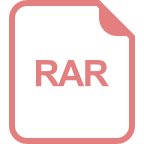
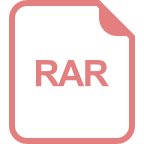
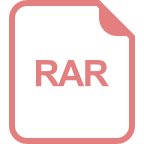
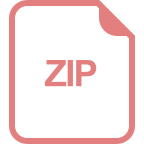
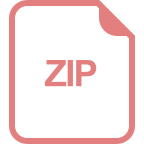
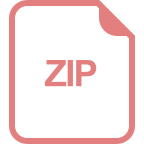
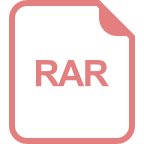
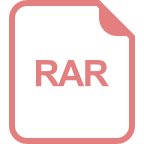
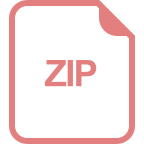
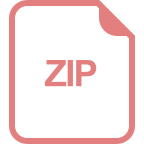