有张带文字的图片,背景颜色会变化。py写一个函数判断 当前屏幕内是否有这样的图片,如果存在返回图像在屏幕上的坐标、图像是否有多个,如果不存在则报错。
时间: 2024-04-29 16:21:14 浏览: 7
这个任务需要使用图像识别技术来完成,可以使用Python的Pillow库和PyAutoGUI库实现。
首先,需要将带文字的图片准备好,并保存为一个文件。然后,可以使用Pillow库加载该图片,并获取其大小和像素数据。
接下来,可以使用PyAutoGUI库的screenshot函数获取当前屏幕的截图,并使用Pillow库加载该截图。然后,可以使用Pillow库的image.search函数在当前屏幕中查找带文字的图片。
如果找到了该图片,则可以获取其在屏幕上的坐标,并使用PyAutoGUI库的click函数点击该图片。如果存在多个相同的图片,则需要进行适当的处理。
如果没有找到该图片,则可以抛出一个异常或返回一个错误信息。
下面是一个可能的实现:
```python
import pyautogui
from PIL import Image
def find_image(filename):
# Load the image file
image = Image.open(filename)
image_width, image_height = image.size
image_data = list(image.getdata())
# Take a screenshot of the screen
screenshot = pyautogui.screenshot()
screenshot_width, screenshot_height = screenshot.size
screenshot_data = list(screenshot.getdata())
# Search for the image in the screenshot
locations = []
for i in range(screenshot_width - image_width):
for j in range(screenshot_height - image_height):
if screenshot_data[j * screenshot_width + i : (j + image_height) * screenshot_width + i] == image_data:
locations.append((i, j))
# Check if the image was found
if not locations:
raise ValueError("Image not found")
# Click on the first location
pyautogui.click(locations[0])
# Return the locations
if len(locations) == 1:
return locations[0], False
else:
return locations, True
```
该函数的参数是带文字的图片的文件名。如果找到了该图片,则返回图像在屏幕上的坐标和一个布尔值,表示是否存在多个相同的图片。如果没有找到该图片,则抛出一个ValueError异常。
可以使用以下代码测试该函数:
```python
try:
location, multiple = find_image("text_image.png")
print(f"Image found at {location}, multiple images: {multiple}")
except ValueError as e:
print(e)
```
该代码会尝试查找名为"text_image.png"的带文字的图片。如果找到了该图片,则会输出其在屏幕上的坐标和一个布尔值,表示是否存在多个相同的图片。如果没有找到该图片,则会输出一个错误信息。
相关推荐
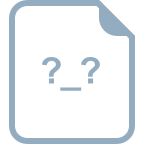
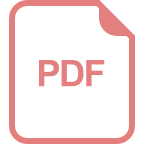














