C语言编程实现用堆排序及快速排序算法,对待用二叉链表建立的二叉排序树进行中序遍历后的结果进行排序并输出排序结果。
时间: 2023-07-20 10:32:38 浏览: 102
好的,我可以帮你解答这个问题。
首先,我需要确认一下你对二叉排序树的概念是否清楚。如果不清楚,简单来说,二叉排序树是一种特殊的二叉树,它的每个节点都满足以下两个条件:
1. 左子树中所有节点的值都小于当前节点的值;
2. 右子树中所有节点的值都大于当前节点的值。
使用二叉排序树进行中序遍历,可以得到一个有序的序列。如果对这个序列进行排序,可以使用堆排序和快速排序算法。下面是使用这两种算法对中序遍历结果进行排序的示例代码:
1. 堆排序算法
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉排序树节点
typedef struct node {
int data; // 节点数据
struct node* left; // 左子树
struct node* right; // 右子树
} Node;
// 插入节点
void insert_node(Node** root, int data) {
if (*root == NULL) {
// 如果根节点为空,新建一个节点
*root = (Node*)malloc(sizeof(Node));
(*root)->data = data;
(*root)->left = NULL;
(*root)->right = NULL;
} else if (data < (*root)->data) {
// 如果插入的值小于根节点的值,插入到左子树中
insert_node(&((*root)->left), data);
} else {
// 如果插入的值大于等于根节点的值,插入到右子树中
insert_node(&((*root)->right), data);
}
}
// 中序遍历
void inorder_traversal(Node* root) {
if (root == NULL) {
return;
}
inorder_traversal(root->left);
printf("%d ", root->data);
inorder_traversal(root->right);
}
// 获取二叉排序树节点个数
int get_node_count(Node* root) {
if (root == NULL) {
return 0;
}
return get_node_count(root->left) + get_node_count(root->right) + 1;
}
// 将二叉排序树中序遍历结果存入数组中
void inorder_to_array(Node* root, int arr[], int* index) {
if (root == NULL) {
return;
}
inorder_to_array(root->left, arr, index);
arr[(*index)++] = root->data;
inorder_to_array(root->right, arr, index);
}
// 交换两个数的值
void swap(int* a, int* b) {
int temp = *a;
*a = *b;
*b = temp;
}
// 调整堆
void adjust_heap(int arr[], int i, int size) {
int lchild = 2 * i + 1;
int rchild = 2 * i + 2;
int max = i;
if (lchild < size && arr[lchild] > arr[max]) {
max = lchild;
}
if (rchild < size && arr[rchild] > arr[max]) {
max = rchild;
}
if (max != i) {
swap(&arr[i], &arr[max]);
adjust_heap(arr, max, size);
}
}
// 堆排序
void heap_sort(int arr[], int size) {
int i;
// 建堆
for (i = size / 2 - 1; i >= 0; i--) {
adjust_heap(arr, i, size);
}
// 排序
for (i = size - 1; i >= 1; i--) {
swap(&arr[0], &arr[i]);
adjust_heap(arr, 0, i);
}
}
// 输出数组
void print_arr(int arr[], int size) {
int i;
for (i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
printf("\n");
}
int main() {
Node* root = NULL;
int arr[100];
int size = 0;
int i;
// 插入节点
insert_node(&root, 5);
insert_node(&root, 2);
insert_node(&root, 6);
insert_node(&root, 0);
insert_node(&root, 3);
insert_node(&root, 9);
insert_node(&root, 1);
insert_node(&root, 7);
insert_node(&root, 4);
insert_node(&root, 8);
// 中序遍历
printf("Inorder traversal:\n");
inorder_traversal(root);
printf("\n");
// 将中序遍历结果存入数组中
size = get_node_count(root);
inorder_to_array(root, arr, &i);
// 堆排序
heap_sort(arr, size);
// 输出排序结果
printf("Sorted array:\n");
print_arr(arr, size);
return 0;
}
```
2. 快速排序算法
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉排序树节点
typedef struct node {
int data; // 节点数据
struct node* left; // 左子树
struct node* right; // 右子树
} Node;
// 插入节点
void insert_node(Node** root, int data) {
if (*root == NULL) {
// 如果根节点为空,新建一个节点
*root = (Node*)malloc(sizeof(Node));
(*root)->data = data;
(*root)->left = NULL;
(*root)->right = NULL;
} else if (data < (*root)->data) {
// 如果插入的值小于根节点的值,插入到左子树中
insert_node(&((*root)->left), data);
} else {
// 如果插入的值大于等于根节点的值,插入到右子树中
insert_node(&((*root)->right), data);
}
}
// 中序遍历
void inorder_traversal(Node* root) {
if (root == NULL) {
return;
}
inorder_traversal(root->left);
printf("%d ", root->data);
inorder_traversal(root->right);
}
// 获取二叉排序树节点个数
int get_node_count(Node* root) {
if (root == NULL) {
return 0;
}
return get_node_count(root->left) + get_node_count(root->right) + 1;
}
// 将二叉排序树中序遍历结果存入数组中
void inorder_to_array(Node* root, int arr[], int* index) {
if (root == NULL) {
return;
}
inorder_to_array(root->left, arr, index);
arr[(*index)++] = root->data;
inorder_to_array(root->right, arr, index);
}
// 交换两个数的值
void swap(int* a, int* b) {
int temp = *a;
*a = *b;
*b = temp;
}
// 快速排序
void quick_sort(int arr[], int left, int right) {
if (left >= right) {
return;
}
int pivot = arr[left];
int i = left + 1;
int j = right;
while (1) {
while (i <= right && arr[i] < pivot) {
i++;
}
while (j >= left + 1 && arr[j] > pivot) {
j--;
}
if (i > j) {
break;
}
swap(&arr[i], &arr[j]);
i++;
j--;
}
swap(&arr[left], &arr[j]);
quick_sort(arr, left, j - 1);
quick_sort(arr, j + 1, right);
}
// 输出数组
void print_arr(int arr[], int size) {
int i;
for (i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
printf("\n");
}
int main() {
Node* root = NULL;
int arr[100];
int size = 0;
int i;
// 插入节点
insert_node(&root, 5);
insert_node(&root, 2);
insert_node(&root, 6);
insert_node(&root, 0);
insert_node(&root, 3);
insert_node(&root, 9);
insert_node(&root, 1);
insert_node(&root, 7);
insert_node(&root, 4);
insert_node(&root, 8);
// 中序遍历
printf("Inorder traversal:\n");
inorder_traversal(root);
printf("\n");
// 将中序遍历结果存入数组中
size = get_node_count(root);
inorder_to_array(root, arr, &i);
// 快速排序
quick_sort(arr, 0, size - 1);
// 输出排序结果
printf("Sorted array:\n");
print_arr(arr, size);
return 0;
}
```
希望这些代码对你有所帮助!
阅读全文
相关推荐









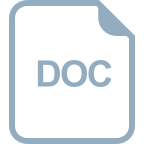







