springboot+mybatisplus实现分页功能,并返回mysql数据库数
时间: 2023-09-08 11:04:55 浏览: 229
### 回答1:
使用 MyBatis-Plus 实现分页功能,可以使用 MyBatis-Plus 的分页插件。
在 Spring Boot 中使用 MyBatis-Plus 分页,需要在配置文件中启用分页插件,例如:
```
mybatis-plus.mapper-locations=classpath:/mapper/*.xml
mybatis-plus.type-aliases-package=com.example.domain
mybatis-plus.configuration.map-underscore-to-camel-case=true
mybatis-plus.configuration.log-impl=org.apache.ibatis.logging.stdout.StdOutImpl
mybatis-plus.configuration.default-fetch-size=100
mybatis-plus.configuration.default-statement-timeout=30
mybatis-plus.configuration.cache-enabled=false
mybatis-plus.configuration.jdbc-type-for-null=NULL
mybatis-plus.configuration.lazy-loading-enabled=true
mybatis-plus.configuration.aggressive-lazy-loading=false
mybatis-plus.configuration.multiple-result-sets-enabled=true
mybatis-plus.configuration.use-generated-keys=false
mybatis-plus.configuration.use-column-label=true
mybatis-plus.configuration.auto-mapping-behavior=FULL
mybatis-plus.configuration.safe-row-bounds-enabled=false
mybatis-plus.configuration.local-cache-scope=SESSION
mybatis-plus.configuration.jdbc-fetch-size=100
mybatis-plus.configuration.max-failures-count=10
mybatis-plus.configuration.call-setters-on-nulls=false
mybatis-plus.configuration.return-instance-for-empty-row=false
mybatis-plus.configuration.map-underscore-to-camel-case=true
mybatis-plus.configuration.use-actual-param-name=true
mybatis-plus.configuration.log-prefix=mp
mybatis-plus.configuration.log-level=debug
mybatis-plus.configuration.force-use-generated-keys=false
mybatis-plus.configuration.aggressive-lazy-loading=false
mybatis-plus.configuration.auto-mapping-behavior=FULL
mybatis-plus.configuration.safe-result-handler-enabled=true
mybatis-plus.configuration.safe-result-handler-enabled=true
mybatis-plus.configuration.auto-mapping-behavior=FULL
mybatis-plus.configuration.default-executor-type=SIMPLE
mybatis-plus.configuration.default-statement-timeout=25
mybatis-plus.configuration.default-fetch-size=100
mybatis-plus.configuration.safe-row
### 回答2:
使用Spring Boot和MyBatis Plus实现分页功能的步骤如下:
1. 在Spring Boot的项目中引入MyBatis Plus的依赖。可以在pom.xml文件中加入如下代码:
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>最新版本</version>
</dependency>
```
注意:需将最新版本替换为实际的MyBatis Plus版本号。
2. 创建实体类。根据数据库中的表结构,创建与之对应的实体类,并在类上使用MyBatis Plus提供的注解@Table来指定对应的数据库表名。
3. 创建Mapper接口。创建一个继承自MyBatis Plus提供的BaseMapper接口的自定义Mapper接口,并使用注解@Mapper来标注该接口。
4. 配置分页插件。在application.properties(或application.yaml)配置文件中添加以下配置:
```properties
# 分页插件配置
mybatis-plus.pagehelper.helper-dialect=mysql
mybatis-plus.pagehelper.reasonable=true
mybatis-plus.pagehelper.support-methods-arguments=true
```
5. 在Service层编写分页查询方法。在Service层的具体方法中,使用MyBatis Plus的Page类来实现分页功能,示例代码如下:
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public Page<User> getUserList(int pageNum, int pageSize) {
Page<User> page = new Page<>(pageNum, pageSize);
QueryWrapper<User> queryWrapper = new QueryWrapper<>();
// 可根据需要添加查询条件
// queryWrapper.eq("name", "xxx");
return userMapper.selectPage(page, queryWrapper);
}
}
```
6. 在Controller层调用分页查询方法。在Controller层中,根据请求参数传入需要的页码和每页数量,调用Service层的分页查询方法,并将结果返回给前端。示例代码如下:
```java
@Controller
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/user/list")
public String getUserList(@RequestParam("pageNum") int pageNum, @RequestParam("pageSize") int pageSize, Model model) {
Page<User> page = userService.getUserList(pageNum, pageSize);
model.addAttribute("userList", page.getRecords());
model.addAttribute("total", page.getTotal());
return "userList"; // 返回到前端页面
}
}
```
以上是使用Spring Boot和MyBatis Plus实现分页功能并返回MySQL数据库中的数据的步骤和示例代码。
### 回答3:
使用Spring Boot集成MyBatis Plus实现分页功能并返回MySQL数据库数据的方法如下:
1. 首先,在pom.xml文件中添加相关依赖:
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>最新版本</version>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-generator</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
</dependencies>
```
2. 创建一个实体类,该实体类对应数据库中的表,并使用MyBatis Plus的注解进行配置,如:
```java
@TableName("user")
public class User {
@TableId(value = "id", type = IdType.AUTO)
private Long id;
@TableField("name")
private String name;
// 省略getter和setter方法
}
```
3. 创建Mapper接口,使用MyBatis Plus提供的BaseMapper接口或者继承BaseMapper接口,如:
```java
public interface UserMapper extends BaseMapper<User> {
}
```
4. 创建Service层接口和实现类,实现分页查询的功能,如:
```java
public interface UserService {
IPage<User> getUserList(int pageNum, int pageSize);
}
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public IPage<User> getUserList(int pageNum, int pageSize) {
Page<User> page = new Page<>(pageNum, pageSize); // 构建分页查询条件
return userMapper.selectPage(page, null); // 进行分页查询
}
}
```
5. 创建Controller层,使用UserService中的方法获取分页数据,并返回给前端,如:
```java
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users")
public IPage<User> getUsers(@RequestParam(defaultValue = "1") int pageNum,
@RequestParam(defaultValue = "10") int pageSize) {
return userService.getUserList(pageNum, pageSize);
}
}
```
6. 最后,配置数据库连接信息,如在application.properties文件中添加以下信息:
```
spring.datasource.url=jdbc:mysql://localhost:3306/database_name
spring.datasource.username=your_username
spring.datasource.password=your_password
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
```
以上步骤完成后,启动Spring Boot应用程序,在浏览器访问`localhost:8080/users`即可获取分页数据,并返回到前端。MySQL数据库中的`database_name`为你所使用的数据库名称,`your_username`为你的数据库用户名,`your_password`为你的数据库密码。
阅读全文
相关推荐
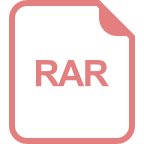
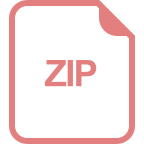
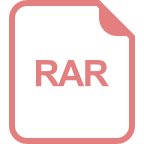

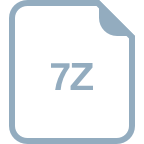
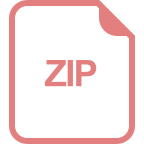
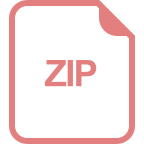
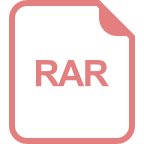
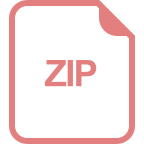
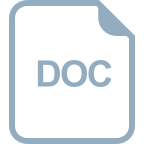
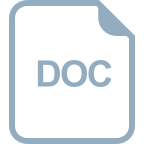
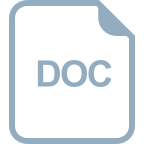


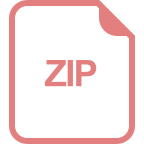
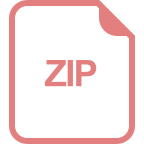
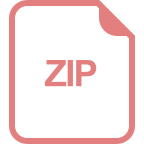
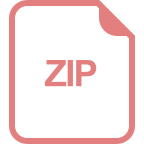