使用Java编写一个加密系统
时间: 2024-06-10 10:09:54 浏览: 20
作为AI语言模型,我可以为您提供一些思路和代码示例,但是我不能实际运行代码。
思路:
1. 设计加密算法。可以选择常用的对称加密算法,如AES、DES等,也可以自行设计简单的加密算法。
2. 设计密钥生成算法。密钥是加密算法中的关键,需要考虑安全性和唯一性。
3. 编写加密和解密方法。加密方法将明文转化为密文,解密方法将密文转化为明文。
4. 编写主函数,读取用户输入的明文或密文,调用加密或解密方法,输出结果。
代码示例:
这里提供一个使用AES对称加密算法的加密系统示例。
```java
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import java.security.SecureRandom;
import java.util.Scanner;
public class EncryptionSystem {
private static final String ALGORITHM = "AES"; // 加密算法
private static final int KEY_SIZE = 128; // 密钥长度
private static final String CHARSET_NAME = "UTF-8"; // 字符集
public static void main(String[] args) throws Exception {
// 生成密钥
SecretKey secretKey = generateKey();
byte[] keyBytes = secretKey.getEncoded();
// 输出密钥
System.out.println("密钥:" + byteArrayToHexString(keyBytes));
// 加密/解密
Scanner scanner = new Scanner(System.in);
System.out.print("请输入明文或密文:");
String input = scanner.nextLine();
if (isHexString(input)) { // 如果是密文
byte[] cipherBytes = hexStringToByteArray(input);
byte[] plainBytes = decrypt(cipherBytes, keyBytes);
String plainText = new String(plainBytes, CHARSET_NAME);
System.out.println("明文:" + plainText);
} else { // 如果是明文
byte[] plainBytes = input.getBytes(CHARSET_NAME);
byte[] cipherBytes = encrypt(plainBytes, keyBytes);
String cipherText = byteArrayToHexString(cipherBytes);
System.out.println("密文:" + cipherText);
}
}
/**
* 生成密钥
*/
private static SecretKey generateKey() throws Exception {
KeyGenerator keyGenerator = KeyGenerator.getInstance(ALGORITHM);
keyGenerator.init(KEY_SIZE, new SecureRandom());
return keyGenerator.generateKey();
}
/**
* 加密
*/
private static byte[] encrypt(byte[] plainBytes, byte[] keyBytes) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, new SecretKeySpec(keyBytes, ALGORITHM));
return cipher.doFinal(plainBytes);
}
/**
* 解密
*/
private static byte[] decrypt(byte[] cipherBytes, byte[] keyBytes) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, new SecretKeySpec(keyBytes, ALGORITHM));
return cipher.doFinal(cipherBytes);
}
/**
* 将字节数组转换为十六进制字符串
*/
private static String byteArrayToHexString(byte[] bytes) {
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
sb.append(String.format("%02X", b));
}
return sb.toString();
}
/**
* 将十六进制字符串转换为字节数组
*/
private static byte[] hexStringToByteArray(String hexString) {
int len = hexString.length();
byte[] bytes = new byte[len / 2];
for (int i = 0; i < len; i += 2) {
bytes[i / 2] = (byte) ((Character.digit(hexString.charAt(i), 16) << 4)
+ Character.digit(hexString.charAt(i + 1), 16));
}
return bytes;
}
/**
* 判断字符串是否为十六进制字符串
*/
private static boolean isHexString(String str) {
return str.matches("[0-9a-fA-F]+");
}
}
```
此示例中,密钥采用默认的128位长度,加密算法采用AES,字符集采用UTF-8。输入明文或密文后,程序会自动判断并调用加密或解密方法,最终输出明文或密文。
相关推荐
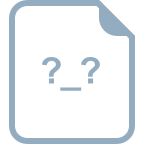
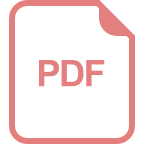














