用Java编写一个复杂的图书管理系统
时间: 2023-09-06 17:13:56 浏览: 173
好的,我可以为您提供一些指导和建议。首先,您需要确定系统的功能需求和设计。以下是一些可能包括在复杂的图书管理系统中的功能:
1. 图书管理:包括添加、删除、修改和搜索图书信息。
2. 用户管理:包括添加、删除、修改和搜索用户信息。
3. 借书和还书:包括借出图书和接收归还的图书,以及管理借阅历史记录。
4. 借阅规则:包括借阅时间、续借次数、罚款和提醒。
5. 权限管理:包括管理员、普通用户和游客的不同权限和功能。
6. 数据统计:包括借阅量、流通率、图书损耗等统计信息。
接下来,您可以开始设计系统的架构和数据模型,以及选择合适的技术栈。Java是一种常用的编程语言,常用的数据库有MySQL、Oracle等。您可以使用Spring框架或其他适合的框架来帮助您构建应用程序。此外,您还可以考虑使用前端框架如React或Angular来构建用户界面。
在实现过程中,您需要注意系统的安全性和稳定性。例如,采用密码加密、访问控制等措施来保护用户隐私和系统数据。同时,您需要进行充分的测试和调试,确保系统能够正常运行并具有合理的性能。
希望这些建议对您有所帮助。如果您有任何进一步的问题或需要更多的帮助,请随时与我联系。
相关问题
java java编写一个图书管理系统
Java是一种面向对象的编程语言,它可以用于开发各种类型的应用程序,包括图书管理系统。下面是一个简单的Java图书管理系统的实现方式:
1. 首先,需要定义一个Book类,该类包含书籍的基本信息,如书名、作者、出版社、ISBN号等。可以使用以下代码定义Book类:
```
public class Book {
private String title;
private String author;
private String publisher;
private String isbn;
public Book(String title, String author, String publisher, String isbn) {
this.title = title;
this.author = author;
this.publisher = publisher;
this.isbn = isbn;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public String getPublisher() {
return publisher;
}
public String getIsbn() {
return isbn;
}
}
```
2. 接下来,需要定义一个BookManager类,该类包含对图书进行管理的方法,如添加图书、删除图书、查找图书等。可以使用以下代码定义BookManager类:
```
import java.util.ArrayList;
import java.util.List;
public class BookManager {
private List<Book> books;
public BookManager() {
books = new ArrayList<>();
}
public void addBook(Book book) {
books.add(book);
}
public void removeBook(Book book) {
books.remove(book);
}
public Book findBookByTitle(String title) {
for (Book book : books) {
if (book.getTitle().equals(title)) {
return book;
}
}
return null;
}
public Book findBookByAuthor(String author) {
for (Book book : books) {
if (book.getAuthor().equals(author)) {
return book;
}
}
return null;
}
public Book findBookByIsbn(String isbn) {
for (Book book : books) {
if (book.getIsbn().equals(isbn)) {
return book;
}
}
return null;
}
}
```
3. 最后,可以编写一个简单的控制台程序来测试图书管理系统。可以使用以下代码实现:
```
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
BookManager manager = new BookManager();
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("请选择操作:");
System.out.println("1. 添加图书");
System.out.println("2. 删除图书");
System.out.println("3. 查找图书");
System.out.println("4. 退出");
int choice = scanner.nextInt();
scanner.nextLine();
switch (choice) {
case 1:
System.out.println("请输入书名:");
String title = scanner.nextLine();
System.out.println("请输入作者:");
String author = scanner.nextLine();
System.out.println("请输入出版社:");
String publisher = scanner.nextLine();
System.out.println("请输入ISBN号:");
String isbn = scanner.nextLine();
Book book = new Book(title, author, publisher, isbn);
manager.addBook(book);
System.out.println("添加成功!");
break;
case 2:
System.out.println("请输入书名:");
title = scanner.nextLine();
book = manager.findBookByTitle(title);
if (book != null) {
manager.removeBook(book);
System.out.println("删除成功!");
} else {
System.out.println("未找到该书!");
}
break;
case 3:
System.out.println("请选择查找方式:");
System.out.println("1. 按书名查找");
System.out.println("2. 按作者查找");
System.out.println("3. 按ISBN号查找");
int searchChoice = scanner.nextInt();
scanner.nextLine();
switch (searchChoice) {
case 1:
System.out.println("请输入书名:");
title = scanner.nextLine();
book = manager.findBookByTitle(title);
if (book != null) {
System.out.println("书名:" + book.getTitle());
System.out.println("作者:" + book.getAuthor());
System.out.println("出版社:" + book.getPublisher());
System.out.println("ISBN号:" + book.getIsbn());
} else {
System.out.println("未找到该书!");
}
break;
case 2:
System.out.println("请输入作者:");
author = scanner.nextLine();
book = manager.findBookByAuthor(author);
if (book != null) {
System.out.println("书名:" + book.getTitle());
System.out.println("作者:" +
使用java编写一个图书管理系统
好的,下面是一个简单的图书管理系统的Java代码示例:
```
import java.util.ArrayList;
import java.util.Scanner;
public class BookManagementSystem {
static Scanner scanner = new Scanner(System.in);
static ArrayList<Book> bookList = new ArrayList<>();
public static void main(String[] args) {
while (true) {
System.out.println("欢迎来到图书管理系统");
System.out.println("1.添加图书");
System.out.println("2.删除图书");
System.out.println("3.查询图书");
System.out.println("4.显示所有图书");
System.out.println("5.退出系统");
int choice = scanner.nextInt();
switch (choice) {
case 1:
addBook();
break;
case 2:
deleteBook();
break;
case 3:
searchBook();
break;
case 4:
displayAllBooks();
break;
case 5:
System.out.println("感谢使用图书管理系统,再见!");
System.exit(0);
default:
System.out.println("无效的选项,请重新选择");
break;
}
}
}
public static void addBook() {
System.out.println("请输入图书编号:");
int id = scanner.nextInt();
System.out.println("请输入图书名称:");
String name = scanner.next();
System.out.println("请输入图书作者:");
String author = scanner.next();
System.out.println("请输入图书价格:");
double price = scanner.nextDouble();
Book book = new Book(id, name, author, price);
bookList.add(book);
System.out.println("添加图书成功!");
}
public static void deleteBook() {
System.out.println("请输入要删除的图书编号:");
int id = scanner.nextInt();
for (int i = 0; i < bookList.size(); i++) {
Book book = bookList.get(i);
if (book.getId() == id) {
bookList.remove(i);
System.out.println("删除图书成功!");
return;
}
}
System.out.println("未找到指定编号的图书");
}
public static void searchBook() {
System.out.println("请输入要查询的图书名称:");
String name = scanner.next();
for (Book book : bookList) {
if (book.getName().equals(name)) {
System.out.println(book);
return;
}
}
System.out.println("未找到指定名称的图书");
}
public static void displayAllBooks() {
for (Book book : bookList) {
System.out.println(book);
}
}
}
class Book {
private int id;
private String name;
private String author;
private double price;
public Book(int id, String name, String author, double price) {
this.id = id;
this.name = name;
this.author = author;
this.price = price;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public String getAuthor() {
return author;
}
public double getPrice() {
return price;
}
@Override
public String toString() {
return "编号:" + id + ",名称:" + name + ",作者:" + author + ",价格:" + price;
}
}
```
这个图书管理系统实现了以下功能:
1. 添加图书
2. 删除图书
3. 查询图书
4. 显示所有图书
你可以根据需要进行修改和扩展。
阅读全文
相关推荐
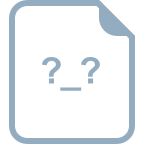
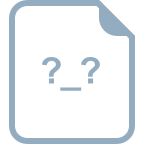
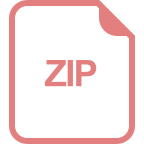













