#include <iostream> class Shape { public: virtual void draw() { std::cout << "Drawing a generic shape." << std::endl; } }; class Circle : public Shape { public: void draw() { std::cout << "Drawing a circle." << std::endl; } }; class Rectangle : public Shape { public: void draw() { std::cout << "Drawing a rectangle." << std::endl; } }; int main() { Shape* s1 = new Circle(); Shape* s2 = new Rectangle(); s1->draw(); s2->draw(); delete s1; delete s2; return 0; } 这段c++代码中,虚函数 draw 为什么不能打印输出 Drawubg a generic shape呢?
时间: 2024-01-09 21:04:30 浏览: 22
在这段代码中,虚函数 draw 在基类 Shape 中被声明,并被派生类 Circle 和 Rectangle 重写。在程序中,我们创建了基类指针 s1 和 s2,并将其指向派生类对象 Circle 和 Rectangle,然后调用它们的 draw 函数。
由于基类指针 s1 和 s2 的类型都是 Shape 类型,所以在调用它们的 draw 函数时,会根据指针类型去调用相应的函数。因为 s1 指向 Circle 对象,s2 指向 Rectangle 对象,所以分别调用的是 Circle 和 Rectangle 类中的 draw 函数。
如果我们想让基类指针 s1 和 s2 调用基类中的 draw 函数,我们可以在基类中将 draw 函数声明为纯虚函数,这样派生类必须实现该函数,否则无法编译通过。或者我们可以在派生类中显式地调用基类的 draw 函数。
修改后的代码如下:
```
#include <iostream>
class Shape {
public:
virtual void draw() = 0; // 声明为纯虚函数
};
class Circle : public Shape {
public:
void draw() {
std::cout << "Drawing a circle." << std::endl;
}
};
class Rectangle : public Shape {
public:
void draw() {
std::cout << "Drawing a rectangle." << std::endl;
}
};
int main() {
Shape* s1 = new Circle();
Shape* s2 = new Rectangle();
s1->draw();
s2->draw();
delete s1;
delete s2;
return 0;
}
```
输出结果为:
```
Drawing a circle.
Drawing a rectangle.
```
相关推荐
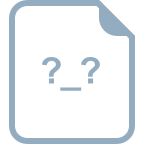














