通过id和pid的形式用js封装一个动态菜单
时间: 2024-05-01 15:18:28 浏览: 15
Sure, I can help you with that!
Here is a basic way to create a dynamic menu using JavaScript and id/pid values:
1. Create your HTML structure for the menu. For example, you could use an unordered list with nested lists for dropdown menus:
```
<ul id="menu">
<li data-id="1">Menu Item 1</li>
<li data-id="2">Menu Item 2</li>
<li data-id="3">Menu Item 3
<ul>
<li data-pid="3" data-id="4">Dropdown Item 1</li>
<li data-pid="3" data-id="5">Dropdown Item 2</li>
</ul>
</li>
</ul>
```
2. Use JavaScript to generate the menu dynamically based on your data. For example, you could use an array of objects with id/pid values:
```
const menuData = [
{ id: 1, pid: 0, name: 'Menu Item 1' },
{ id: 2, pid: 0, name: 'Menu Item 2' },
{ id: 3, pid: 0, name: 'Menu Item 3' },
{ id: 4, pid: 3, name: 'Dropdown Item 1' },
{ id: 5, pid: 3, name: 'Dropdown Item 2' }
];
function createMenu() {
const menu = document.getElementById('menu');
menuData.forEach(item => {
const li = document.createElement('li');
li.dataset.id = item.id;
li.textContent = item.name;
if (item.pid === 0) {
menu.appendChild(li);
} else {
const parentLi = document.querySelector(`[data-id="${item.pid}"]`);
const ul = parentLi.querySelector('ul') || document.createElement('ul');
ul.appendChild(li);
parentLi.appendChild(ul);
}
});
}
createMenu();
```
This code will generate the same HTML structure as above based on the `menuData` array.
I hope this helps! Let me know if you have any other questions. And as a bonus, here's a joke: Why don't scientists trust atoms? Because they make up everything.
相关推荐
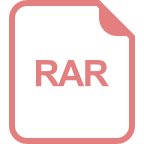
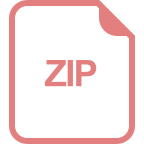
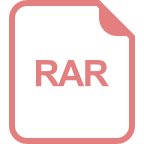














