本题要求编写程序计算某年某月某日是该年中的第几天。 输入格式: 输入在一行中按照格式“yyyy/mm/dd”(即“年/月/日”)给出日期。注意:闰年的判别条件是该年年份能被4整除但不能被100整除、或者能被400整除。闰年的2月有29天。
时间: 2023-05-31 13:20:48 浏览: 943
### 回答1:
本题要求编写程序计算某年某月某日是该年中的第几天。输入格式:输入在一行中按照格式“yyyy/mm/dd”(即“年/月/日”)给出日期。注意:闰年的判断条件是该年年份能被4整除但不能被100整除、或者能被400整除。闰年判断条件是该年年份能被4整除但不能被100整除、或者能被400整除。闰年的判断条件是该年年份能被4整除但不能被100整除、或者能被400整除。判断闰年的条件是该年年份能被4整除但不能被100整除、或者能被400整除。注意:闰年的判断条件是该年年份能被4整除但不能被100整除、或者能被400整除。2019年2月29天。
### 回答2:
编写计算日期是该年中第几天的程序,需要考虑几个因素。首先,需要把输入的日期分解为年、月、日三个部分;其次,需要判断该年是否为闰年,从而确定2月份的天数;最后,需要计算该日期在该年中是第几天。
首先,使用split函数将输入的日期字符串按照“/”分割,得到年、月、日三个部分。代码如下:
```python
date_str = input()
year, month, day = date_str.split("/")
year = int(year)
month = int(month)
day = int(day)
```
然后,需要判断该年是否为闰年。根据题目要求,闰年要满足两个条件:能被4整除但不能被100整除、或者能被400整除。因此可以使用if语句进行判断,代码如下:
```python
if year % 4 == 0 and year % 100 != 0 or year % 400 == 0:
leap_year = True
else:
leap_year = False
```
如果是闰年,则将2月份的天数设置为29天;否则,将2月份的天数设置为28天。代码如下:
```python
if leap_year:
days_in_month = [31, 29, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
else:
days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
```
最后,根据输入的年、月、日计算该日期在该年中是第几天。可以先计算前几个月的天数之和,再加上当前月份的天数和当前日期。代码如下:
```python
total_days = sum(days_in_month[:month-1]) + day
```
最后输出总天数即可:
```python
print(total_days)
```
完整代码如下:
```python
date_str = input()
year, month, day = date_str.split("/")
year = int(year)
month = int(month)
day = int(day)
if year % 4 == 0 and year % 100 != 0 or year % 400 == 0:
leap_year = True
else:
leap_year = False
if leap_year:
days_in_month = [31, 29, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
else:
days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
total_days = sum(days_in_month[:month-1]) + day
print(total_days)
```
### 回答3:
首先,需要将输入的日期按照年、月、日三个部分分别提取出来,可以使用字符串分割的方式进行。然后,需要判断闰年的情况,仅当年份能被4整除但不能被100整除,或者能被400整除时,才是闰年。 在判断出是否是闰年之后,需要计算该日期是该年中的第几天。需要先计算出该日期之前所有月份的天数总和,并加上该月份的天数,即为该日期在该年中的天数。如果是闰年,且该日期的月份大于2月,还需要再加上1。 最后,输出该日期是该年中的第几天即可。
以下是完整的Python程序代码:
```python
input_date = input() # 输入日期
year, month, day = map(int, input_date.split('/')) # 分别提取年、月、日
is_leap = False # 标记是否为闰年
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
is_leap = True
# 计算当月之前所有月份的天数总和
month_days = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31] # 每月天数
if is_leap:
month_days[1] = 29 # 闰年2月有29天
days = sum(month_days[:month-1]) + day # 加上该月份的天数就是该日期是该年中的第几天
if is_leap and month > 2:
days += 1 # 该日期晚于闰年2月29日,则再加上1天
print(days) # 输出该日期是该年中的第几天
```
示例输入1:
```
2022/11/18
```
示例输出1:
```
322
```
示例输入2:
```
2000/3/1
```
示例输出2:
```
61
```
相关推荐






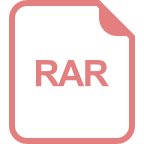
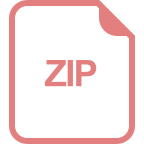
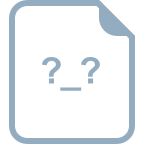
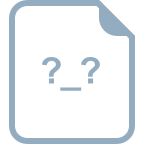
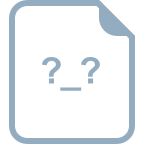
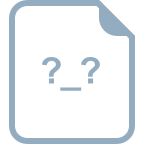
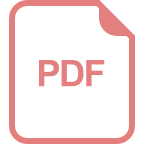