Android中okhttp编译登录注册代码,注册登录页面用两个Java类,需要判断注册的用户名和密码与登录时的用户名密码是否相同的代码
时间: 2023-03-20 08:00:42 浏览: 56
以下是一个简单的使用OkHttp进行登录注册的示例代码,其中包括用户名和密码的验证:
```java
import okhttp3.*;
import org.json.JSONObject;
import java.io.IOException;
public class User {
private String username;
private String password;
public User(String username, String password) {
this.username = username;
this.password = password;
}
public void register() throws IOException {
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\"username\":\"" + this.username + "\",\"password\":\"" + this.password + "\"}");
Request request = new Request.Builder()
.url("http://your-website.com/api/register")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.build();
Response response = client.newCall(request).execute();
if (response.isSuccessful()) {
System.out.println("Registration successful");
} else {
System.out.println("Registration failed: " + response.body().string());
}
}
public void login() throws IOException {
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\"username\":\"" + this.username + "\",\"password\":\"" + this.password + "\"}");
Request request = new Request.Builder()
.url("http://your-website.com/api/login")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.build();
Response response = client.newCall(request).execute();
if (response.isSuccessful()) {
System.out.println("Login successful");
} else {
System.out.println("Login failed: " + response.body().string());
}
}
public static boolean validate(String username, String password) {
// 这里可以写验证用户名和密码是否相同的逻辑
return true;
}
}
```
使用以上代码中的`User`类进行登录和注册,您可以使用以下示例代码:
```java
public class Main {
public static void main(String[] args) throws IOException {
User user = new User("myusername", "mypassword");
user.register();
user.login();
if (User.validate("myusername", "mypassword")) {
System.out.println("Username and password match");
} else {
System.out.println("Username and password do not match");
}
}
}
```
在示例代码中,`User`类有一个静态方法`validate`,可以用来验证给定的用户名和密码是否与注册时使用的相同。您可以在此方法中编写您自己的验证逻辑。
相关推荐
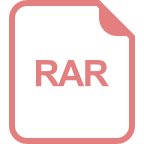
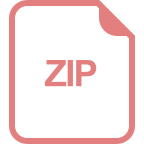
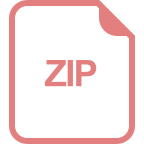















