vscode 插件开发 选中文本
时间: 2023-06-23 11:08:54 浏览: 287
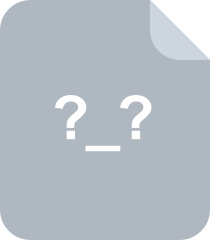
VS Code 中文插件
要在 VS Code 插件中选择文本,可以使用以下代码:
```
// 获取当前选中的文本
const editor = vscode.window.activeTextEditor;
const selection = editor.selection;
const selectedText = editor.document.getText(selection);
// 将光标移动到选中文本的末尾
editor.selection = new vscode.Selection(selection.end, selection.end);
```
这段代码将获取当前活动编辑器中的选中文本,并将光标移动到选中文本的末尾。你可以在插件中使用这段代码来处理选中文本。
阅读全文
相关推荐
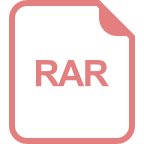
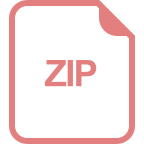
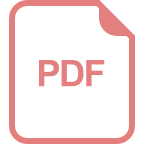
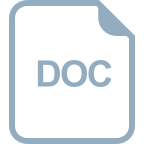
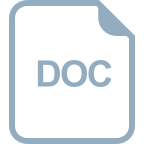



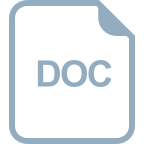





