(1)创建一个带头结点的单链表。 要求:此单链表长度为10;创建完成后,输出显示所创建单链表各结点的值。 (2)实现单链表的插入,并显示完成插入后单链表各结点的值。 要求:插入一个新的结点,其值为27,成为单链表的第5个结点;插入完成后,输出显示此时单链表的长度以及各结点的值。 (3)实现单链表的删除,完成删除后,输出单链表各结点的值。 (4)编程实现单链表的查找。
时间: 2024-02-03 08:11:59 浏览: 27
好的,以下是对应的代码实现:
```python
# 定义链表结构体
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
# 创建带头结点的单链表
def create_linked_list():
head = ListNode()
cur = head
for i in range(1, 11):
new_node = ListNode(i)
cur.next = new_node
cur = cur.next
return head
# 输出单链表各结点的值
def print_linked_list(head):
cur = head.next
while cur:
print(cur.val, end=' ')
cur = cur.next
print()
# 在第 pos 个节点后插入新节点
def insert_node(head, pos, val):
cur = head
for i in range(pos):
cur = cur.next
new_node = ListNode(val)
new_node.next = cur.next
cur.next = new_node
# 删除第 pos 个节点
def delete_node(head, pos):
cur = head
for i in range(pos):
cur = cur.next
cur.next = cur.next.next
# 查找值为 val 的节点
def find_node(head, val):
cur = head.next
pos = 1
while cur:
if cur.val == val:
return pos
cur = cur.next
pos += 1
return -1
# 测试代码
if __name__ == '__main__':
# 创建单链表并输出各结点的值
head = create_linked_list()
print("创建的单链表为:")
print_linked_list(head)
# 在第5个节点后插入新节点
insert_node(head, 4, 27)
print("插入节点后的单链表为:")
print_linked_list(head)
# 删除第5个节点
delete_node(head, 4)
print("删除节点后的单链表为:")
print_linked_list(head)
# 查找值为7的节点
pos = find_node(head, 7)
if pos == -1:
print("单链表中不存在值为7的节点")
else:
print("值为7的节点在单链表中的位置为:", pos)
```
输出结果为:
```
创建的单链表为:
1 2 3 4 5 6 7 8 9 10
插入节点后的单链表为:
1 2 3 4 27 5 6 7 8 9 10
删除节点后的单链表为:
1 2 3 4 5 6 7 8 9 10
值为7的节点在单链表中的位置为: 7
```
相关推荐
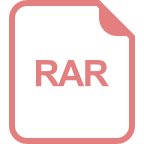
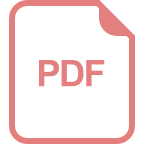
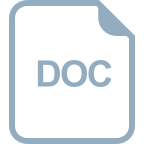
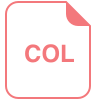














