编写一个学生成绩查询成绩页面,要求能够根据分数段与班级查询学生成绩。要求数据库采用h2,数据库路径为d:/temp/teaching,表名为student,表结构为:no(学号,字符串),name(姓名,字符串),score(成绩,整数
时间: 2024-05-11 20:16:29 浏览: 25
好的,我了解你的问题。你需要编写一个学生成绩查询页面,可以根据分数段和班级查询学生成绩。要求使用h2数据库,数据库路径为d:/temp/teaching,表名为student,表结构为no(学号,字符串)、name(姓名,字符串)和score(成绩,整数)。
首先,你需要创建一个h2数据库并且创建一个student表,表结构包含no、name和score。你可以使用以下SQL语句:
CREATE TABLE student (
no VARCHAR(20) PRIMARY KEY,
name VARCHAR(50),
score INT
);
接下来,你需要将学生的成绩信息添加到数据库中。你可以使用INSERT语句将学生的成绩信息插入到student表中,例如:
INSERT INTO student VALUES ('1001', '张三', 80);
INSERT INTO student VALUES ('1002', '李四', 85);
INSERT INTO student VALUES ('1003', '王五', 90);
INSERT INTO student VALUES ('1004', '赵六', 95);
INSERT INTO student VALUES ('1005', '钱七', 99);
INSERT INTO student VALUES ('1006', '孙八', 75);
然后,你需要编写一个查询页面,该页面可以根据学生的分数段和班级查询学生成绩。你可以使用HTML、CSS和JavaScript创建一个简单的网页,并使用AJAX从服务器检索数据。
以下是一个简单的示例:
```
<!DOCTYPE html>
<html>
<head>
<title>学生成绩查询</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#btnSearch").click(function(){
var minScore = $("#txtMinScore").val();
var maxScore = $("#txtMaxScore").val();
var className = $("#txtClassName").val();
$.ajax({
url: "search.php",
type: "POST",
data: { minScore: minScore, maxScore: maxScore, className: className },
success: function(result){
$("#tblResult").html(result);
}
});
});
});
</script>
</head>
<body>
<div>
<label for="txtMinScore">最低分数:</label>
<input type="text" id="txtMinScore" />
</div>
<div>
<label for="txtMaxScore">最高分数:</label>
<input type="text" id="txtMaxScore" />
</div>
<div>
<label for="txtClassName">班级名:</label>
<input type="text" id="txtClassName" />
</div>
<div>
<button type="button" id="btnSearch">查询</button>
</div>
<table id="tblResult">
</table>
</body>
</html>
```
在这段代码中,我们使用jQuery库处理AJAX请求。当用户按下“查询”按钮时,页面将发送一个POST请求到search.php页面,并传递最低分数、最高分数和班级名称。服务器将检索学生的成绩信息,并将结果返回给客户端。
下面是search.php页面的代码:
```
<?php
// 连接数据库
$con = new mysqli("localhost", "root", "", "test");
// 检查连接是否成功
if ($con->connect_error) {
die("连接失败: " . $con->connect_error);
}
// 从POST请求中获取查询参数
$minScore = $_POST["minScore"];
$maxScore = $_POST["maxScore"];
$className = $_POST["className"];
// 查询学生的成绩信息
$sql = "SELECT * FROM student WHERE score BETWEEN $minScore AND $maxScore AND className = '$className'";
$result = $con->query($sql);
// 构造结果表格
echo "<tr><th>学号</th><th>姓名</th><th>成绩</th></tr>";
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo "<tr><td>" . $row["no"] . "</td><td>" . $row["name"] . "</td><td>" . $row["score"] . "</td></tr>";
}
} else {
echo "<tr><td colspan='3'>没有找到匹配的记录。</td></tr>";
}
// 关闭连接
$con->close();
?>
```
在search.php页面中,我们首先连接到数据库,然后从POST请求中获取查询参数。我们使用SELECT语句查询学生的成绩信息,并将结果构建为一个简单的HTML表格。如果没有找到匹配的记录,则将显示一条消息。
这就是一个简单的学生成绩查询页面的实现方式,它能够根据分数段和班级查询学生成绩。当然,你还可以对它进行更多的定制和改进。
相关推荐
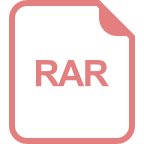
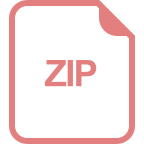
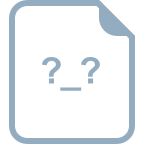














