C++:有一个师傅进程和三个学徒进程,每个学徒连续不断地组装产品,做一个产品需要A、B、C三种零件,这三个学徒分别掌握有A零件、B零件、C零件多个。 要求完成以下功能: (1)师傅源源不断地供应上述三种零件,但他每次只将其中的两种零件放在桌子上(所放零件由rand % 3函数生成的0-2之间的随机数决定)。 (2)具有另一零件的学徒就可以组装产品,且做完后给师傅发信号,然后师傅再拿出两种零件放在桌上。 (3)每次只能有一个进程放入或取出零件。 (4)利用同步与互斥的相关知识编写仿真系统实现以上过程,要能够清晰表达产品生产的全过程。
时间: 2024-02-15 09:03:57 浏览: 126
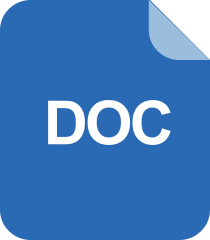
一个C++程序
这是一个经典的生产者消费者问题,可以使用互斥锁和条件变量来解决。
首先,需要定义三个互斥锁来分别保护A、B、C三种零件的数量,以及一个互斥锁来保护师傅放置零件的操作。每个学徒进程需要等待至少一种零件可用,才能进行组装并通知师傅放置新的两种零件。
以下是一个可能的实现:
```
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define NUM_APPRENTICES 3
#define NUM_PARTS 3
pthread_mutex_t mutex_parts[NUM_PARTS];
pthread_mutex_t mutex_master;
pthread_cond_t cond_apprentices[NUM_APPRENTICES];
int num_parts[NUM_PARTS] = {0};
int num_products = 0;
void produce_parts() {
// 生产随机两种零件
int part1 = rand() % NUM_PARTS;
int part2 = rand() % NUM_PARTS;
while (part2 == part1) {
part2 = rand() % NUM_PARTS;
}
pthread_mutex_lock(&mutex_master);
printf("Master: placing parts %d and %d on the table.\n", part1, part2);
num_parts[part1]++;
num_parts[part2]++;
pthread_mutex_unlock(&mutex_master);
}
void *apprentice(void *arg) {
int part = *(int *)arg;
while (1) {
pthread_mutex_lock(&mutex_parts[part]);
while (num_parts[part] < 1) {
pthread_cond_wait(&cond_apprentices[part], &mutex_parts[part]);
}
num_parts[part]--;
pthread_mutex_unlock(&mutex_parts[part]);
pthread_mutex_lock(&mutex_parts[(part+1)%NUM_PARTS]);
while (num_parts[(part+1)%NUM_PARTS] < 1) {
pthread_cond_wait(&cond_apprentices[(part+1)%NUM_PARTS], &mutex_parts[(part+1)%NUM_PARTS]);
}
num_parts[(part+1)%NUM_PARTS]--;
pthread_mutex_unlock(&mutex_parts[(part+1)%NUM_PARTS]);
printf("Apprentice %d: assembling product %d.\n", part, num_products);
num_products++;
pthread_mutex_lock(&mutex_master);
pthread_cond_signal(&cond_apprentices[part]);
pthread_mutex_unlock(&mutex_master);
}
}
int main() {
srand(time(NULL));
pthread_mutex_init(&mutex_master, NULL);
for (int i = 0; i < NUM_PARTS; i++) {
pthread_mutex_init(&mutex_parts[i], NULL);
}
for (int i = 0; i < NUM_APPRENTICES; i++) {
pthread_cond_init(&cond_apprentices[i], NULL);
}
pthread_t apprentices[NUM_APPRENTICES];
int apprentice_args[NUM_APPRENTICES] = {0, 1, 2};
for (int i = 0; i < NUM_APPRENTICES; i++) {
pthread_create(&apprentices[i], NULL, apprentice, &apprentice_args[i]);
}
while (1) {
produce_parts();
usleep(500000);
}
return 0;
}
```
在上面的代码中,师傅进程用 `produce_parts` 函数来模拟放置两种零件的操作,每次放置后,会通知等待相应零件的学徒进程。每个学徒进程在等待到相应两种零件可用后,会进行组装,并通知师傅进程放置新的两种零件。具体的同步和互斥操作使用了互斥锁和条件变量来实现。
阅读全文
相关推荐
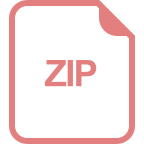
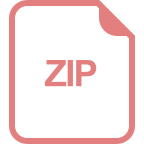
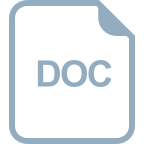
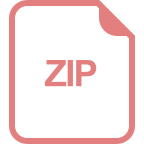
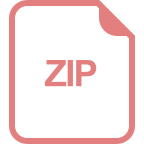
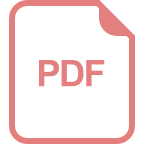
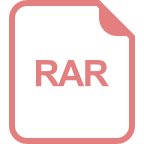
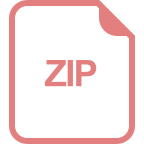
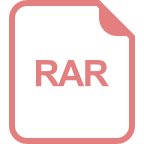
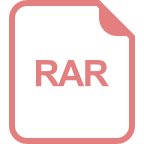
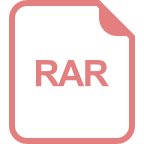
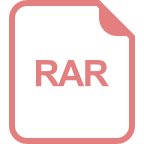
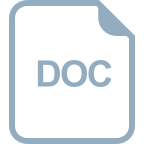
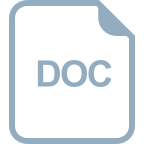