定义一个长度为N的整型有序数组(有序是指数组中元素已按从小到大或从大到小排列),请定义折半查找函数,并在c语言中主函数中调用该折半查找算法实现查找功能,并统计查找过程中数据元素比较次数。
时间: 2024-03-25 11:38:12 浏览: 69
好的,我可以为您解答该问题。
以下是折半查找函数的实现:
```c
int binary_search(int arr[], int n, int x, int* comparisons) {
int low = 0, high = n - 1;
while (low <= high) {
int mid = (low + high) / 2;
(*comparisons)++;
if (arr[mid] == x) {
return mid;
} else if (arr[mid] < x) {
low = mid + 1;
} else {
high = mid - 1;
}
}
return -1;
}
```
该函数接收三个参数:一个有序整型数组 `arr`,数组长度 `n`,要查找的元素 `x`,以及一个 `comparisons` 指针,用于记录比较次数。
在函数中,我们初始化 `low` 和 `high` 分别为数组的第一个和最后一个元素的下标。接着,我们在一个 while 循环中执行以下步骤:
- 计算中间元素的下标 `mid`。
- 比较 `arr[mid]` 和 `x` 的大小。
- 如果 `arr[mid]` 等于 `x`,则返回 `mid`。
- 如果 `arr[mid]` 小于 `x`,则说明要查找的元素在右半部分,将 `low` 更新为 `mid + 1`。
- 如果 `arr[mid]` 大于 `x`,则说明要查找的元素在左半部分,将 `high` 更新为 `mid - 1`。
如果 while 循环结束时还没有找到要查找的元素,那么说明该元素不存在于数组中,返回 -1。
在主函数中,我们可以这样调用折半查找函数:
```c
int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int n = sizeof(arr) / sizeof(arr[0]);
int x = 5;
int comparisons = 0;
int index = binary_search(arr, n, x, &comparisons);
if (index != -1) {
printf("%d found at index %d\n", x, index);
} else {
printf("%d not found\n", x);
}
printf("Number of comparisons: %d\n", comparisons);
```
在上面的代码中,我们定义了一个有序数组 `arr`,数组长度 `n`,要查找的元素 `x`,以及一个变量 `comparisons` 用于记录比较次数。
我们调用 `binary_search` 函数,并传递数组、数组长度、要查找的元素以及 `comparisons` 的地址作为参数。如果函数返回的下标不是 -1,则说明找到了要查找的元素,输出该元素在数组中的下标;否则,输出未找到该元素的提示。最后,输出比较次数。
总的来说,折半查找算法的时间复杂度为 O(log n),比较次数取决于要查找的元素在数组中的位置。如果要查找的元素在数组的中间位置,则只需要一次比较即可找到;如果要查找的元素在数组的两端,则需要多次比较。
阅读全文
相关推荐





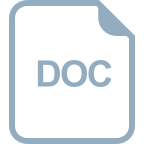







