网页授权前端获取认证code的vue代码
时间: 2024-06-09 12:11:26 浏览: 97
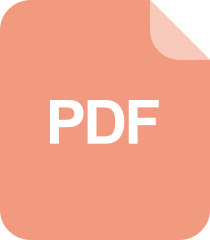
详解Vue微信授权登录前后端分离较为优雅的解决方案
以下是使用Vue.js和Axios库实现网页授权前端获取认证code的代码示例:
```html
<!-- index.html -->
<template>
<div>
<button @click="getAuthCode">获取授权</button>
</div>
</template>
<script>
import axios from 'axios';
export default {
methods: {
getAuthCode() {
const appId = 'your_app_id';
const redirectUri = encodeURIComponent('http://your_redirect_uri');
const scope = 'snsapi_userinfo';
const state = '123';
const url = `https://open.weixin.qq.com/connect/oauth2/authorize?appid=${appId}&redirect_uri=${redirectUri}&response_type=code&scope=${scope}&state=${state}#wechat_redirect`;
window.location.href = url; // 使用window.location.href进行页面跳转
}
}
}
</script>
```
在上述代码中,我们首先定义了一个按钮,当用户点击该按钮时,会调用 `getAuthCode` 方法。该方法会构造一个跳转链接,其中包含了我们的App ID、重定向URI、授权作用域和自定义状态等参数。最后,我们使用 `window.location.href` 进行页面跳转。
注意,此处的 `redirectUri` 参数需要进行URL编码。如果你使用的是Webpack或者Vue CLI等工具,可以使用 `encodeURIComponent` 方法进行编码。
在实际开发中,我们可能需要将获取的 `code` 参数发送给后端服务器进行进一步的处理。我们可以使用Axios库向后端发送HTTP请求,如下所示:
```html
<!-- index.html -->
<template>
<div>
<button @click="getAuthCode">获取授权</button>
</div>
</template>
<script>
import axios from 'axios';
export default {
methods: {
getAuthCode() {
const appId = 'your_app_id';
const redirectUri = encodeURIComponent('http://your_redirect_uri');
const scope = 'snsapi_userinfo';
const state = '123';
const url = `https://open.weixin.qq.com/connect/oauth2/authorize?appid=${appId}&redirect_uri=${redirectUri}&response_type=code&scope=${scope}&state=${state}#wechat_redirect`;
window.location.href = url;
// 在页面跳转后,获取code参数并发送给后端服务器
const code = new URLSearchParams(window.location.search).get('code');
if (code) {
axios.get('http://your_backend_url', {
params: { code: code }
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
}
}
}
}
</script>
```
在上述代码中,我们监听了页面跳转后的URL,并提取了其中的 `code` 参数。如果 `code` 参数存在,我们使用Axios库向后端发送GET请求,并将 `code` 参数作为参数发送。在请求成功后,我们可以在控制台中打印出响应数据。
当然,实际开发中还需要考虑一些安全性和稳定性的问题,例如对URL进行校验、处理异常情况等等。以上代码仅供参考,具体实现需要根据项目需求进行调整。
阅读全文
相关推荐
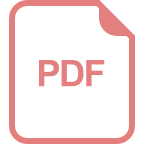
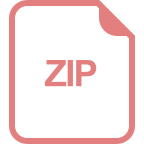
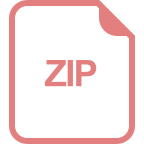
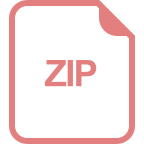
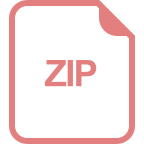
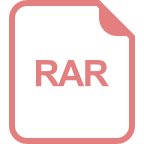
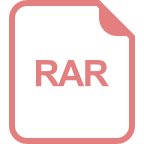
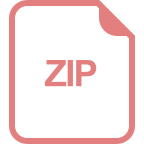
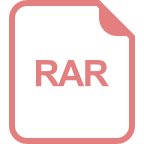
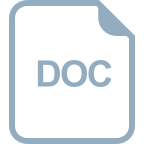
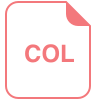




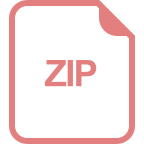
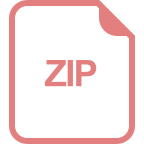
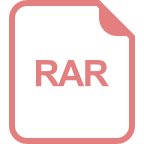