写一个mm32F0144C6p对于W25Q128的驱动代码
时间: 2024-03-12 21:46:44 浏览: 143
以下是一个简单的mm32F0144C6p对于W25Q128的SPI驱动代码,供参考:
```c
#include "mm32F0144.h"
#include "W25Q128.h"
#define SPIx SPI1
#define SPIx_RCC RCC_APB2Periph_SPI1
#define SPIx_GPIO_RCC RCC_AHBPeriph_GPIOA
#define SPIx_GPIO GPIOA
#define SPIx_CS GPIO_Pin_4
#define SPIx_SCK GPIO_Pin_5
#define SPIx_MISO GPIO_Pin_6
#define SPIx_MOSI GPIO_Pin_7
void SPIx_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_AHBPeriphClockCmd(SPIx_GPIO_RCC, ENABLE);
GPIO_InitStructure.GPIO_Pin = SPIx_CS | SPIx_SCK | SPIx_MISO | SPIx_MOSI;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(SPIx_GPIO, &GPIO_InitStructure);
}
void SPIx_Config(void)
{
SPI_InitTypeDef SPI_InitStructure;
RCC_APB2PeriphClockCmd(SPIx_RCC, ENABLE);
SPI_InitStructure.SPI_Direction = SPI_Direction_2Lines_FullDuplex;
SPI_InitStructure.SPI_Mode = SPI_Mode_Master;
SPI_InitStructure.SPI_DataSize = SPI_DataSize_8b;
SPI_InitStructure.SPI_CPOL = SPI_CPOL_Low;
SPI_InitStructure.SPI_CPHA = SPI_CPHA_1Edge;
SPI_InitStructure.SPI_NSS = SPI_NSS_Soft;
SPI_InitStructure.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_4;
SPI_InitStructure.SPI_FirstBit = SPI_FirstBit_MSB;
SPI_InitStructure.SPI_CRCPolynomial = 7;
SPI_Init(SPIx, &SPI_InitStructure);
SPI_Cmd(SPIx, ENABLE);
}
void W25Q128_CS(uint8_t state)
{
if (state == 0)
GPIO_ResetBits(SPIx_GPIO, SPIx_CS);
else
GPIO_SetBits(SPIx_GPIO, SPIx_CS);
}
uint8_t SPIx_WriteReadByte(uint8_t byte)
{
while (SPI_I2S_GetFlagStatus(SPIx, SPI_I2S_FLAG_TXE) == RESET);
SPI_I2S_SendData(SPIx, byte);
while (SPI_I2S_GetFlagStatus(SPIx, SPI_I2S_FLAG_RXNE) == RESET);
return SPI_I2S_ReceiveData(SPIx);
}
void W25Q128_Init(void)
{
SPIx_GPIO_Config();
SPIx_Config();
}
void W25Q128_WriteEnable(void)
{
W25Q128_CS(0);
SPIx_WriteReadByte(W25X_WriteEnable);
W25Q128_CS(1);
}
void W25Q128_WaitForWriteEnd(void)
{
uint8_t flashstatus = 0;
W25Q128_CS(0);
SPIx_WriteReadByte(W25X_ReadStatusReg);
do
{
flashstatus = SPIx_WriteReadByte(W25X_Dummy_Byte);
} while ((flashstatus & W25X_WIP_Flag) == SET);
W25Q128_CS(1);
}
void W25Q128_EraseChip(void)
{
W25Q128_WriteEnable();
W25Q128_CS(0);
SPIx_WriteReadByte(W25X_ChipErase);
W25Q128_CS(1);
W25Q128_WaitForWriteEnd();
}
void W25Q128_WritePage(uint8_t* pBuffer, uint32_t WriteAddr, uint16_t NumByteToWrite)
{
W25Q128_WriteEnable();
W25Q128_CS(0);
SPIx_WriteReadByte(W25X_PageProgram);
SPIx_WriteReadByte((WriteAddr & 0xFF0000) >> 16);
SPIx_WriteReadByte((WriteAddr & 0xFF00) >> 8);
SPIx_WriteReadByte(WriteAddr & 0xFF);
while (NumByteToWrite--)
{
SPIx_WriteReadByte(*pBuffer);
pBuffer++;
}
W25Q128_CS(1);
W25Q128_WaitForWriteEnd();
}
void W25Q128_ReadData(uint8_t* pBuffer, uint32_t ReadAddr, uint16_t NumByteToRead)
{
W25Q128_CS(0);
SPIx_WriteReadByte(W25X_ReadData);
SPIx_WriteReadByte((ReadAddr & 0xFF0000) >> 16);
SPIx_WriteReadByte((ReadAddr & 0xFF00) >> 8);
SPIx_WriteReadByte(ReadAddr & 0xFF);
while (NumByteToRead--)
{
*pBuffer = SPIx_WriteReadByte(W25X_Dummy_Byte);
pBuffer++;
}
W25Q128_CS(1);
}
```
这里只是一个简单的示例代码,实际应用还需要根据具体情况进行修改。
阅读全文
相关推荐













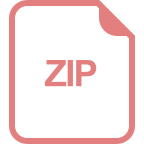
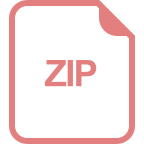
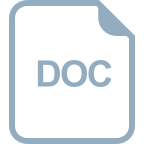