cv2.createCLAHE
时间: 2023-07-02 16:06:47 浏览: 174
cv2.createCLAHE是OpenCV中的一个函数,用于创建一个局部对比度自适应直方图均衡化(CLAHE)对象。CLAHE是一种将图像分成小块并对每个块进行直方图均衡化的技术,可以在增强图像细节的同时避免过度增强噪声。使用cv2.createCLAHE函数可以设置CLAHE对象的各种参数,例如块大小、直方图bin数等。CLAHE对象可以用于对图像进行直方图均衡化,从而改善图像对比度。下面是一个示例代码:
```
import cv2
# 读取图像
img = cv2.imread('image.jpg', 0)
# 创建CLAHE对象
clahe = cv2.createCLAHE(clipLimit=2.0, tileGridSize=(8,8))
# 对图像进行直方图均衡化
img_clahe = clahe.apply(img)
# 显示原图和处理后的图像
cv2.imshow('Original Image', img)
cv2.imshow('CLAHE Image', img_clahe)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个示例中,我们使用cv2.createCLAHE函数创建了一个CLAHE对象,并设置了clipLimit和tileGridSize参数。然后,我们使用CLAHE对象对图像进行直方图均衡化,并显示原始图像和处理后的图像。
相关问题
cv2.createclahe函数中cliplimit参数解释
cv2.createclahe函数中的cliplimit参数是指对比度限制,它控制了CLAHE算法中直方图像的剪裁程度,即在计算直方图生成LUT(look-up table)时,超过cliplimit的像素数会被截断,这有助于避免一些异常像素值的影响,同时也能加快处理速度。
用Numpy实现的CLAHE函数,输入是彩图,不要用cv2.createCLAHE!!!
CLAHE(Contrast Limited Adaptive Histogram Equalization)是一种非线性映射方式,用于增强图像的对比度。下面是用Numpy实现的CLAHE函数,可以输入彩图。
```python
import numpy as np
def clahe(img, clip_limit=2.0, grid_size=(8, 8)):
"""
Contrast Limited Adaptive Histogram Equalization (CLAHE)
:param img: input image, should be a numpy array with shape (H, W, C)
:param clip_limit: threshold for contrast limiting
:param grid_size: size of grid for histogram equalization
:return: CLAHE-enhanced image
"""
# convert image to LAB color space
lab_img = cv2.cvtColor(img, cv2.COLOR_RGB2LAB)
clahe_img = np.zeros_like(lab_img)
# apply CLAHE to each channel of LAB image separately
for i in range(3):
clahe_img[..., i] = clahe_channel(lab_img[..., i], clip_limit, grid_size)
# convert back to RGB color space
clahe_img = cv2.cvtColor(clahe_img, cv2.COLOR_LAB2RGB)
return clahe_img
def clahe_channel(channel, clip_limit=2.0, grid_size=(8, 8)):
"""
Apply CLAHE to a single channel
:param channel: input channel, should be a numpy array with shape (H, W)
:param clip_limit: threshold for contrast limiting
:param grid_size: size of grid for histogram equalization
:return: CLAHE-enhanced channel
"""
h, w = channel.shape
# compute histogram of image
hist, bins = np.histogram(channel.flatten(), 256, [0, 256])
# compute cumulative distribution function (CDF) of histogram
cdf = hist.cumsum()
cdf = (cdf / cdf[-1]) * 255.0
# compute equalized image using CDF
equalized = np.interp(channel.flatten(), bins[:-1], cdf)
equalized = equalized.reshape((h, w))
# compute contrast limited equalization
grid_h, grid_w = grid_size
gh = int(np.ceil(h / grid_h))
gw = int(np.ceil(w / grid_w))
for i in range(grid_h):
for j in range(grid_w):
# extract grid from equalized image
grid = equalized[i*gh:(i+1)*gh, j*gw:(j+1)*gw]
# compute histogram of grid
hist, bins = np.histogram(grid.flatten(), 256, [0, 256])
# compute cumulative distribution function (CDF) of histogram
cdf = hist.cumsum()
cdf = (cdf / cdf[-1]) * 255.0
# clip CDF to contrast limit
cdf = np.clip(cdf, 0, clip_limit * gw * gh / 256.0)
# compute equalized grid using clipped CDF
equalized_grid = np.interp(grid.flatten(), bins[:-1], cdf)
equalized_grid = equalized_grid.reshape(grid.shape)
# insert equalized grid into output image
clahe_img[i*gh:(i+1)*gh, j*gw:(j+1)*gw] = equalized_grid
return clahe_img
```
这个函数首先将输入的彩图转换为LAB色彩空间,然后分别对L、A、B三个通道应用CLAHE算法,最后再将LAB图像转换回RGB色彩空间。CLAHE算法的核心是对图像分成若干个网格,然后在每个网格中执行直方图均衡化。为了避免增强噪声,CLAHE算法还引入了一个对比度限制的机制,具体来说就是对每个网格的直方图进行裁剪,使得直方图的范围不超过一个阈值。这里的阈值是通过clip_limit参数控制的。
阅读全文
相关推荐
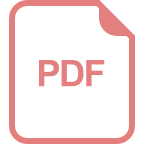
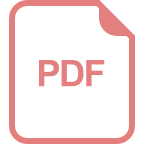
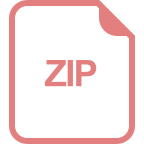












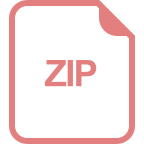