写一个单片机以太网客户端和服务器的函数
时间: 2024-02-03 10:13:51 浏览: 118
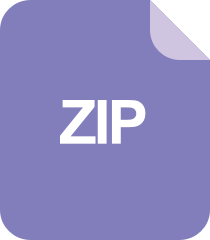
以太网模块 W5500服务器 1对2客户端 STM32单片机测试例程源码.zip

这里提供一个简单的基于STM32单片机的以太网客户端和服务器的函数示例。
客户端函数:
```c
#include <stdio.h>
#include <string.h>
#include "stm32f10x.h"
#include "lwip/opt.h"
#include "lwip/arch.h"
#include "lwip/api.h"
#include "lwip/sys.h"
#include "netif/etharp.h"
// 客户端IP地址
#define CLIENT_IP_ADDR "192.168.1.100"
// 服务器IP地址
#define SERVER_IP_ADDR "192.168.1.200"
// 服务器端口号
#define SERVER_PORT 8888
// 客户端主函数
void client_main(void)
{
err_t err;
struct netconn *conn;
struct netbuf *buf;
char *data;
// 创建一个TCP连接
conn = netconn_new(NETCONN_TCP);
if (conn != NULL)
{
// 设置客户端IP地址和端口号
ip_addr_t addr;
err = netconn_bind(conn, &addr, 0);
if (err == ERR_OK)
{
// 设置服务器IP地址和端口号
err = netconn_connect(conn, IP_ADDR_ANY, SERVER_PORT);
if (err == ERR_OK)
{
// 向服务器发送数据
data = "Hello, server!";
buf = netbuf_new();
netbuf_alloc(buf, strlen(data));
memcpy(netbuf_data(buf), data, strlen(data));
netconn_send(conn, buf);
netbuf_delete(buf);
// 接收服务器的响应
err = netconn_recv(conn, &buf);
if (err == ERR_OK)
{
netbuf_data(buf, &data, &len);
printf("Received data: %s\n", data);
netbuf_delete(buf);
}
}
}
netconn_close(conn);
netconn_delete(conn);
}
}
```
服务器函数:
```c
#include <stdio.h>
#include <string.h>
#include "stm32f10x.h"
#include "lwip/opt.h"
#include "lwip/arch.h"
#include "lwip/api.h"
#include "lwip/sys.h"
#include "netif/etharp.h"
// 服务器IP地址
#define SERVER_IP_ADDR "192.168.1.200"
// 监听端口号
#define LISTEN_PORT 8888
// 服务器主函数
void server_main(void)
{
err_t err;
struct netconn *conn, *newconn;
struct netbuf *buf;
char *data;
u16_t len;
// 创建一个TCP连接
conn = netconn_new(NETCONN_TCP);
if (conn != NULL)
{
// 设置服务器IP地址和端口号
err = netconn_bind(conn, IP_ADDR_ANY, LISTEN_PORT);
if (err == ERR_OK)
{
// 监听客户端连接请求
netconn_listen(conn);
do
{
// 等待客户端连接
err = netconn_accept(conn, &newconn);
if (err == ERR_OK)
{
// 接收客户端发送的数据
err = netconn_recv(newconn, &buf);
if (err == ERR_OK)
{
netbuf_data(buf, &data, &len);
printf("Received data: %s\n", data);
netbuf_delete(buf);
// 向客户端发送响应数据
data = "Hello, client!";
buf = netbuf_new();
netbuf_alloc(buf, strlen(data));
memcpy(netbuf_data(buf), data, strlen(data));
netconn_send(newconn, buf);
netbuf_delete(buf);
}
netconn_close(newconn);
netconn_delete(newconn);
}
} while (err == ERR_OK);
}
netconn_close(conn);
netconn_delete(conn);
}
}
```
这些代码只是示例,需要根据具体的硬件平台和操作系统进行修改和适配。此外,还需要配置网络接口、IP地址、子网掩码、默认网关等网络参数。
阅读全文
相关推荐
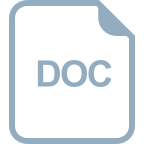
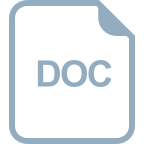
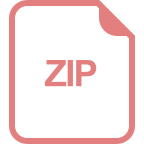
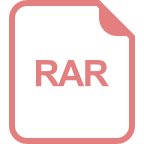
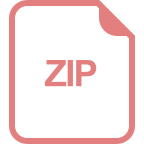
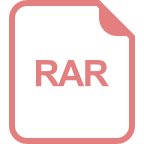
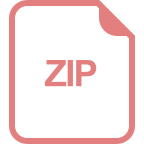
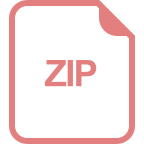
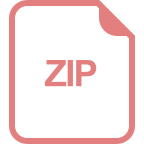
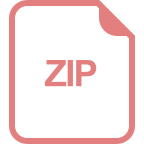
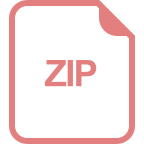
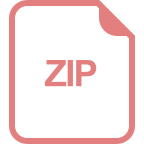
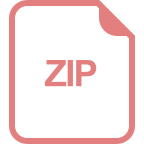
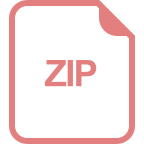
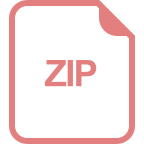
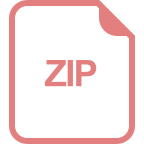
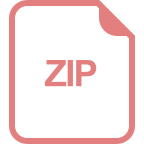
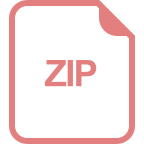