Python爬京东多个商品评论
时间: 2023-08-07 10:24:22 浏览: 58
要爬取京东多个商品评论,可以使用Python中的requests和BeautifulSoup库。
首先,需要获取商品的ID,可以在京东商品详情页面的URL中找到,例如:https://item.jd.com/100010224756.html,其中的“100010224756”就是商品ID。
然后,可以使用requests库发送GET请求获取商品评论页面的HTML代码,例如:
```
import requests
product_id = '100010224756'
url = f'https://club.jd.com/comment/productPageComments.action?productId={product_id}&score=0&sortType=5&page=0&pageSize=10'
response = requests.get(url)
html = response.text
```
其中,score表示评分,0表示全部评分,sortType表示排序方式,5表示按照时间倒序,page表示页码,pageSize表示每页显示评论数。
接着,可以使用BeautifulSoup库解析HTML代码,获取评论内容和评分等信息,例如:
```
from bs4 import BeautifulSoup
soup = BeautifulSoup(html, 'html.parser')
comments = soup.select('.comment-con')
for comment in comments:
content = comment.select('.comment-con .comment-content')[0].text.strip()
score = comment.select('.comment-star .star')[0].get('class')[1][-1]
print(content, score)
```
其中,`.comment-con`表示评论内容的标签,`.comment-content`表示评论内容的子标签,`.comment-star .star`表示评分的标签,`get('class')[1][-1]`表示获取评分的分数。
最后,可以将上述代码封装成函数,循环调用获取多个商品的评论,例如:
```
def get_comments(product_id):
url = f'https://club.jd.com/comment/productPageComments.action?productId={product_id}&score=0&sortType=5&page=0&pageSize=10'
response = requests.get(url)
html = response.text
soup = BeautifulSoup(html, 'html.parser')
comments = soup.select('.comment-con')
results = []
for comment in comments:
content = comment.select('.comment-con .comment-content')[0].text.strip()
score = comment.select('.comment-star .star')[0].get('class')[1][-1]
results.append({'content': content, 'score': score})
return results
product_ids = ['100010224756', '100010224757', '100010224758']
for product_id in product_ids:
comments = get_comments(product_id)
print(comments)
```
阅读全文
相关推荐
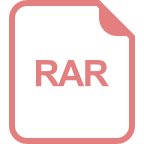
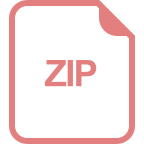
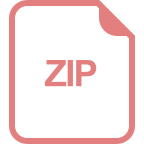
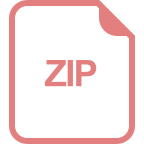
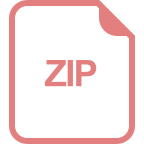
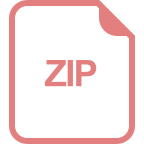
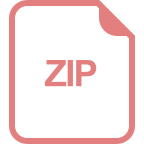
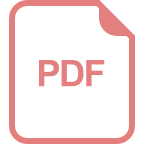
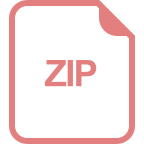
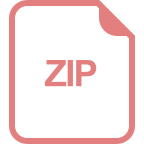
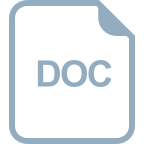
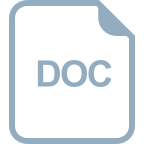


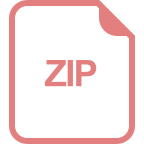
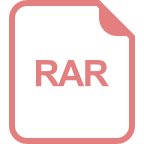

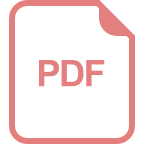