爬虫京东商品信息连接mysql。mysql代码和python代码
时间: 2024-02-04 22:02:18 浏览: 86
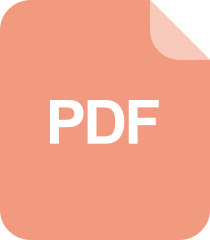
Python爬取当当、京东、亚马逊图书信息代码实例
好的,以下是将爬取到的京东商品信息存入MySQL数据库的Python代码:
```python
import requests
from bs4 import BeautifulSoup
import pymysql
url = 'https://search.jd.com/Search?keyword=python&enc=utf-8'
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36 Edge/16.16299'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
items = soup.select('.gl-item')
# 连接 MySQL 数据库
conn = pymysql.connect(
host='localhost',
user='root',
password='your_password',
db='your_database',
charset='utf8mb4'
)
cursor = conn.cursor()
# 遍历商品列表,插入数据库
for item in items:
name = item.select('.p-name a em')[0].text.strip()
price = item.select('.p-price i')[0].text.strip()
comment_count = item.select('.p-commit a')[0].text.strip()
# 插入数据到 MySQL 数据库
sql = "INSERT INTO product(name, price, comment_count) VALUES (%s, %s, %s)"
cursor.execute(sql, (name, price, comment_count))
conn.commit()
# 关闭数据库连接
cursor.close()
conn.close()
```
这个程序会爬取关键词为“python”的商品信息,并将商品名称、价格和评论数插入到名为“product”的MySQL表中。您需要将代码中的“your_password”和“your_database”替换为您自己的MySQL密码和数据库名。需要注意的是,在执行SQL语句时,我们使用了参数化查询,这可以有效地防止SQL注入攻击。
以下是创建MySQL表的SQL代码:
```mysql
CREATE TABLE `product` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(255) NOT NULL,
`price` decimal(10,2) NOT NULL,
`comment_count` int(11) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
```
这个表包含了三个字段,分别是id(主键)、name(商品名称)、price(商品价格)和comment_count(评论数)。请根据自己的需求修改表名和字段。
阅读全文
相关推荐
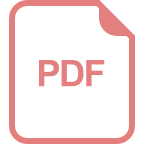
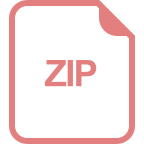















