用java写校园导航系统
时间: 2023-11-08 09:03:55 浏览: 42
好的,下面是一个简单的校园导航系统的Java代码实现,供您参考:
```java
import java.util.*;
// 地点类,表示校园内的一个地点
class Place {
String name; // 地点名称
int x, y; // 地点坐标
public Place(String name, int x, int y) {
this.name = name;
this.x = x;
this.y = y;
}
}
// 边类,表示两个地点之间的道路
class Edge {
Place from, to; // 起点和终点
int length; // 长度
public Edge(Place from, Place to, int length) {
this.from = from;
this.to = to;
this.length = length;
}
}
// 图类,表示整个校园地图
class Graph {
ArrayList<Place> places = new ArrayList<>(); // 地点列表
ArrayList<Edge> edges = new ArrayList<>(); // 道路列表
// 添加地点
public void addPlace(String name, int x, int y) {
places.add(new Place(name, x, y));
}
// 添加道路
public void addEdge(String from, String to, int length) {
Place p1 = findPlace(from);
Place p2 = findPlace(to);
edges.add(new Edge(p1, p2, length));
}
// 查找地点
private Place findPlace(String name) {
for (Place p : places) {
if (p.name.equals(name)) {
return p;
}
}
return null;
}
// 获取两个地点之间的距离
public int getDistance(String from, String to) {
Place p1 = findPlace(from);
Place p2 = findPlace(to);
for (Edge e : edges) {
if (e.from == p1 && e.to == p2) {
return e.length;
}
}
return -1;
}
// 获取最短路径
public ArrayList<Place> getShortestPath(String from, String to) {
Place start = findPlace(from); // 起点
Place end = findPlace(to); // 终点
HashMap<Place, Integer> dist = new HashMap<>(); // 距离表
HashMap<Place, Place> prev = new HashMap<>(); // 前驱表
HashSet<Place> visited = new HashSet<>(); // 已访问集合
PriorityQueue<Place> pq = new PriorityQueue<>(new Comparator<Place>() {
public int compare(Place p1, Place p2) {
return dist.get(p1) - dist.get(p2);
}
}); // 优先队列
// 初始化距离表和前驱表
for (Place p : places) {
dist.put(p, Integer.MAX_VALUE);
prev.put(p, null);
}
dist.put(start, 0);
// 将起点加入优先队列
pq.offer(start);
// 进行Dijkstra算法
while (!pq.isEmpty()) {
Place curr = pq.poll();
if (visited.contains(curr)) {
continue;
}
visited.add(curr);
for (Edge e : edges) {
if (e.from == curr) {
Place next = e.to;
int newDist = dist.get(curr) + e.length;
if (newDist < dist.get(next)) {
dist.put(next, newDist);
prev.put(next, curr);
pq.offer(next);
}
}
}
}
// 构造最短路径
ArrayList<Place> path = new ArrayList<>();
Place curr = end;
while (curr != null) {
path.add(curr);
curr = prev.get(curr);
}
Collections.reverse(path);
return path;
}
}
// 校园导航系统
class CampusNavigationSystem {
Graph graph = new Graph(); // 校园地图
// 初始化校园地图
public void init() {
graph.addPlace("图书馆", 0, 0);
graph.addPlace("教学楼", 100, 0);
graph.addPlace("实验楼", 200, 0);
graph.addPlace("食堂", 0, 100);
graph.addPlace("体育馆", 100, 100);
graph.addPlace("行政楼", 200, 100);
graph.addEdge("图书馆", "教学楼", 50);
graph.addEdge("教学楼", "实验楼", 100);
graph.addEdge("图书馆", "食堂", 30);
graph.addEdge("食堂", "体育馆", 70);
graph.addEdge("体育馆", "行政楼", 80);
graph.addEdge("行政楼", "教学楼", 50);
}
// 根据地点名称获取坐标
private int[] getCoords(String name) {
int[] coords = new int[2];
for (Place p : graph.places) {
if (p.name.equals(name)) {
coords[0] = p.x;
coords[1] = p.y;
}
}
return coords;
}
// 显示地图
public void showMap() {
System.out.println("校园地图:");
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
String name = "";
int x = j * 100 + 50;
int y = i * 100 + 50;
for (Place p : graph.places) {
if (p.x == x && p.y == y) {
name = p.name;
}
}
System.out.printf("%-10s", name);
}
System.out.println();
}
}
// 显示最短路径
public void showShortestPath(String from, String to) {
ArrayList<Place> path = graph.getShortestPath(from, to);
System.out.printf("从%s到%s的最短路径:\n", from, to);
for (int i = 0; i < path.size(); i++) {
System.out.printf("%s(%d,%d)", path.get(i).name, path.get(i).x, path.get(i).y);
if (i < path.size() - 1) {
int dist = graph.getDistance(path.get(i).name, path.get(i + 1).name);
System.out.printf("->(%d)->", dist);
}
}
System.out.println();
}
}
// 测试
public class Test {
public static void main(String[] args) {
CampusNavigationSystem sys = new CampusNavigationSystem();
sys.init();
sys.showMap();
sys.showShortestPath("图书馆", "行政楼");
}
}
```
以上代码实现了一个简单的校园导航系统,包括地点类、边类、图类和校园导航系统类。其中,地点类表示一个校园内的地点,边类表示两个地点之间的道路,图类表示整个校园地图,校园导航系统类实现了校园地图的初始化、地图展示和最短路径导航功能。
在上面的代码中,我们使用了Dijkstra算法求解最短路径,用优先队列实现了算法的优化。在展示最短路径时,我们先通过getShortestPath方法获取最短路径的Place列表,然后根据Place列表和道路长度计算出完整的最短路径,并将其输出到控制台上。
希望以上代码能够对您编写校园导航系统有所帮助。如果您有其他问题或疑问,欢迎继续提问。
相关推荐













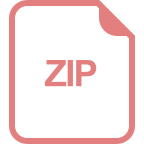