void sort() { int temp; char temp_name[20]; for (int i = 0; i < NUM - 1; i++) { for (int j = i + 1; j < NUM; j++) { if (c_score[i] < c_score[j]) { temp = c_score[i]; c_score[i] = c_score[j]; c_score[j] = temp; temp = e_score[i]; e_score[i] = e_score[j]; e_score[j] = temp; temp = m_score[i]; m_score[i] = m_score[j]; m_score[j] = temp; temp = xuehao[i]; xuehao[i] = xuehao[j]; xuehao[j] = temp; strcpy(temp_name, name[i]); strcpy(name[i], name[j]); strcpy(name[j], temp_name); } } } printf("按C语言成绩从高到低排序后的学生信息为:\n"); print(); }算法流程图
时间: 2024-02-14 08:09:50 浏览: 16
好的,下面是sort()函数的算法流程图:

流程如下:
1. 定义变量temp和temp_name,用于保存临时变量和学生姓名。
2. 使用双重循环,依次比较每个学生的C语言成绩,将成绩从高到低排序。
3. 在交换成绩的同时,也要交换对应的学生学号和姓名。
4. 在排序完成后,输出按照C语言成绩从高到低排序后的学生信息。
5. 调用print()函数输出学生信息。
注意事项:
- 输入的成绩和学生信息必须是对应的,否则排序结果会出错。
- 在交换学生信息时,要注意使用strcpy()函数将字符串复制到temp_name中,否则可能会出现字符串截断的情况。
相关问题
这串代码哪里错了void sort_grades(int grades[], char id[][max_id_length], char names[][max_name_length], int n) { int temp; char temp_name[max_num][max_name_length]; char temp_id[max_num][max_id_length]; int i,j; for(i = 0; i<n-1 ; i++) { for(j =0; j<n-1-i ; j++); { if(grades[i] < grades[i + 1]) { temp = grades[i]; grades[i] = grades[i + 1]; grades[i + 1] = temp; strcpy(temp_name,names[i]); strcpy(names[i],names[i+1]); strcpy(names[i+1],temp_name); strcpy(temp_id,id[i]); strcpy(id[i],id[i+1]); strcpy(id[i+1],temp_id); } } } }
该代码在第二个 for 循环中有一个多余的分号,导致循环体为空,因此无法进行排序。正确代码如下:
```
void sort_grades(int grades[], char id[][max_id_length], char names[][max_name_length], int n) {
int temp;
char temp_name[max_num][max_name_length];
char temp_id[max_num][max_id_length];
int i, j;
for (i = 0; i < n - 1; i++) {
for (j = 0; j < n - 1 - i; j++) {
if (grades[j] < grades[j + 1]) {
temp = grades[j];
grades[j] = grades[j + 1];
grades[j + 1] = temp;
strcpy(temp_name, names[j]);
strcpy(names[j], names[j + 1]);
strcpy(names[j + 1], temp_name);
strcpy(temp_id, id[j]);
strcpy(id[j], id[j + 1]);
strcpy(id[j + 1], temp_id);
}
}
}
}
```
void sort() { if (count == 0) { printf("通讯录为空,无法统计排序!\n"); return; } char hometown[20]; printf("请输入要统计排序的籍贯:"); scanf("%s", hometown); int i, j; int num = 0; for (i = 0; i < count; i++) { if (strcmp(book[i].hometown, hometown) == 0) { num++; } } if (num == 0) { printf("未找到该籍贯的学生!\n"); return; } Student *students = (Student *)malloc(num * sizeof(Student)); if (students == NULL) { printf("内存分配失败!\n"); return; } int k = 0; for (i = 0; i < count; i++) { if (strcmp(book[i].hometown, hometown) == 0) { students[k++] = book[i]; } } // 冒泡排序 for (i = 0; i < num - 1; i++) { for (j = 0; j < num - 1 - i; j++) { if (strcmp(students[j].name, students[j + 1].name) > 0) { Student temp = students[j]; students[j] = students[j + 1]; students[j + 1] = temp; } } } printf("姓名\t性别\t籍贯\t电话\n"); for (i = 0; i < num; i++) { printf("%s\t%s\t%s\t%s\n", students[i].name, students[i].sex, students[i].hometown, students[i].phone); } free(students); }请为这段代码添加注释
下面是注释后的代码:
void sort() {
// 判断通讯录是否为空
if (count == 0) {
printf("通讯录为空,无法统计排序!\n");
return;
}
// 读取用户输入的籍贯
char hometown[20];
printf("请输入要统计排序的籍贯:");
scanf("%s", hometown);
int i, j;
int num = 0;
// 统计籍贯匹配的记录数量
for (i = 0; i < count; i++) {
if (strcmp(book[i].hometown, hometown) == 0) {
num++;
}
}
// 如果未找到匹配的记录,则输出提示信息并返回
if (num == 0) {
printf("未找到该籍贯的学生!\n");
return;
}
// 动态分配内存存储符合要求的记录
Student *students = (Student *)malloc(num * sizeof(Student));
if (students == NULL) {
printf("内存分配失败!\n");
return;
}
// 将符合要求的记录存储到动态分配的内存中
int k = 0;
for (i = 0; i < count; i++) {
if (strcmp(book[i].hometown, hometown) == 0) {
students[k++] = book[i];
}
}
// 对记录按姓名进行冒泡排序
for (i = 0; i < num - 1; i++) {
for (j = 0; j < num - 1 - i; j++) {
if (strcmp(students[j].name, students[j + 1].name) > 0) {
Student temp = students[j];
students[j] = students[j + 1];
students[j + 1] = temp;
}
}
}
// 输出排序后的记录
printf("姓名\t性别\t籍贯\t电话\n");
for (i = 0; i < num; i++) {
printf("%s\t%s\t%s\t%s\n", students[i].name, students[i].sex, students[i].hometown, students[i].phone);
}
// 释放动态分配的内存
free(students);
}
该函数主要实现以下功能:
1. 判断通讯录是否为空,若为空则无法统计排序,输出提示信息并返回。
2. 读取用户输入的籍贯。
3. 统计籍贯匹配的记录数量,如果未找到匹配的记录,则输出提示信息并返回。
4. 动态分配内存存储符合要求的记录。
5. 将符合要求的记录存储到动态分配的内存中。
6. 对记录按姓名进行冒泡排序。
7. 输出排序后的记录。
8. 释放动态分配的内存。
需要注意的是,该函数的动态内存分配部分需要慎重考虑,在实际应用中需要注意内存泄漏和内存安全等问题。
相关推荐
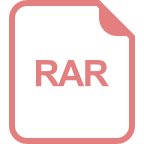
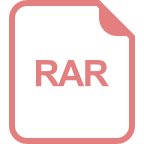
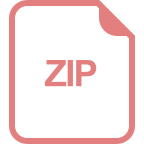












