怎么通过Java将Excel导入或导出数据库
时间: 2024-01-10 20:02:41 浏览: 31
你可以使用 Java 中的 Apache POI 库来读取和写入 Excel 文件,以及使用 JDBC API 将数据插入或提取到数据库中。下面是一个简单的示例代码,将 Excel 数据导入到 MySQL 数据库中。
1. 首先,你需要下载并导入 Apache POI 和 MySQL JDBC 驱动程序到你的项目中。
2. 创建一个 Excel 文件并填充数据,例如:
```
姓名 年龄 性别
张三 25 男
李四 30 女
```
3. 使用以下代码读取 Excel 文件并将数据插入到 MySQL 数据库中:
```java
import java.io.File;
import java.io.FileInputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
public class ExcelToDatabase {
public static void main(String[] args) throws Exception {
// 指定 Excel 文件路径
String excelFilePath = "path/to/excel/file.xlsx";
// 加载 MySQL JDBC 驱动程序
Class.forName("com.mysql.jdbc.Driver");
// 连接到 MySQL 数据库
String url = "jdbc:mysql://localhost:3306/mydatabase";
String username = "username";
String password = "password";
Connection conn = DriverManager.getConnection(url, username, password);
// 准备插入 SQL 语句
String insertSql = "INSERT INTO mytable (name, age, gender) VALUES (?, ?, ?)";
PreparedStatement pstmt = conn.prepareStatement(insertSql);
// 读取 Excel 文件
FileInputStream fis = new FileInputStream(new File(excelFilePath));
Workbook workbook = WorkbookFactory.create(fis);
Sheet sheet = workbook.getSheetAt(0);
// 遍历 Excel 表格并插入到数据库中
for (Row row : sheet) {
Cell nameCell = row.getCell(0);
Cell ageCell = row.getCell(1);
Cell genderCell = row.getCell(2);
String name = nameCell.getStringCellValue();
int age = (int) ageCell.getNumericCellValue();
String gender = genderCell.getStringCellValue();
pstmt.setString(1, name);
pstmt.setInt(2, age);
pstmt.setString(3, gender);
pstmt.executeUpdate();
}
// 关闭资源
workbook.close();
fis.close();
pstmt.close();
conn.close();
}
}
```
这段代码使用 Apache POI 库读取 Excel 文件,使用 JDBC API 将数据插入到 MySQL 数据库中。你可以根据你的需求进行修改和定制。
相关推荐
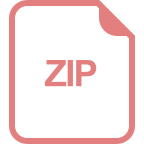














