帮我写一段简单的用基础函数实现的比较低阶的NTRU数字签名的MATLAB代码
时间: 2024-05-01 11:17:33 浏览: 26
好的,我可以为您编写NTRU数字签名的MATLAB代码。以下是一段简单的代码,它使用基础函数实现NTRU数字签名:
```
% NTRU数字签名
% 生成密钥对
N = 503; % NTRU代数,N必须是质数
p = 3; % 多项式系数应该是 +-1, 0 之一,p表示系数的范围,可以为任意质数
q = 5; % 对p取完后,mod q 运算来得到最终明文
f = 3; % 多项式f中非零系数的数目,满足1<=f<=(N+1)/2
g = 2; % 多项式g中非零系数的数目,满足1<=g<=(N+1)/2
p_tmp = (p-1)/2; % 求(p-1)/2的值
ghat = generate_ghat(g, p_tmp, N); % 生成多项式ghat
fhat = generate_fhat(f, g, p_tmp, ghat, N); % 生成多项式fhat
h = inv_mod_p(fhat, ghat, p, N); % 生成私钥h
publickey = {h, g, p, q, N}; % 生成公钥
% 签名过程
plaintext = 'Hello, World!'; % 明文消息
sig = sign_message(plaintext, publickey); % 给消息进行签名
% 验证签名
isvalid = verify_signature(plaintext, sig, publickey); % 验证签名是否有效
disp(isvalid); % 输出结果
function res = generate_ghat(g, p_tmp, N)
% 生成多项式ghat
h = floor(N / 2); % 生成值在[-h, h]的随机整数
ghat = 1 + poly_rand([-h, h], g-1);
while (run_gcd(ghat, [1, zeros(1, N-g), -1], N) ~= 1 || mod_p(ghat, 2, N) == 0 || compute_ones(ghat) ~= g || compute_norm(ghat, p_tmp, N) > h)
ghat = 1 + poly_rand([-h, h], g-1);
end
res = ghat;
end
function res = generate_fhat(f, g, p_tmp, ghat, N)
% 生成多项式fhat
h = floor(N / 2); % 生成值在[-h, h]的随机整数
fhat = poly_rand([-h, h], f-1);
while (run_gcd(fhat, ghat, N) ~= 1 || compute_ones(fhat) ~= f || compute_norm(fhat, p_tmp, N) > h)
fhat = poly_rand([-h, h], f-1);
end
res = fhat;
end
function res = inv_mod_p(fhat, ghat, p, N)
% 生成私钥h
[gcd, x, y] = run_xgcd(fhat, ghat, N);
if (length(fhat) ~= length(ghat))
if (length(fhat) > length(ghat))
ghat = [ghat zeros(1, length(fhat) - length(ghat))];
else
fhat = [fhat zeros(1, length(ghat) - length(fhat))];
end
end
if (mod_p(x, p, N) ~= 1)
x = N - x;
end
h = mod_p(x * fhat, p, N);
res = h;
end
function res = sign_message(plaintext, publickey)
% 给消息进行签名
h = publickey{1};
g = publickey{2};
p = publickey{3};
q = publickey{4};
N = publickey{5};
x = floor(N / 2); % 生成值在[-x, x]的随机整数
r = poly_rand([-x, x], N-1);
e = poly_rand([-x, x], N-1);
s = mod_p(((g * r + h * e)/p) + str2num(dec2base(hash(plaintext), q)), q, N);
res = {r, s};
end
function res = verify_signature(plaintext, sig, publickey)
% 验证签名是否有效
r = sig{1};
s = sig{2};
h = publickey{1};
g = publickey{2};
p = publickey{3};
q = publickey{4};
N = publickey{5};
t = mod_p((s * p - h * str2num(dec2base(hash(plaintext), q)))/g, q, N);
if (isequal(r, run_mod(g * t, N)))
res = true;
else
res = false;
end
end
function res = hash(plaintext)
% 哈希函数
res = sum(double(plaintext));
end
function res = compute_ones(poly)
% 计算多项式中1的个数
res = sum(poly == 1);
end
function res = compute_norm(poly, p_tmp, N)
% 计算多项式的p范数
tmp1 = run_mod(p_tmp, N-1) * sum(abs(poly).^p_tmp);
norm = floor(tmp1 / N);
tmp2 = tmp1 - norm * N;
if (tmp2 > (N - 1)/2)
res = N - tmp2;
else
res = tmp2;
end
end
function res = mod_p(poly, p, N)
% 对多项式进行p模运算
res = cellfun(@(x) mod_p(x, p, N), num2cell(poly));
end
function res = run_mod(poly, N)
% 对多项式进行模运算
res = cellfun(@(x) mod(x, N), num2cell(poly));
end
function res = run_gcd(poly1, poly2, N)
% 求多项式的最大公约数
res = run_mod(poly1, N);
while (length(poly2) > 0)
[quo, rem] = run_div(res, poly2);
res = poly2;
poly2 = rem;
end
end
function res = run_xgcd(poly1, poly2, N)
% 求多项式的扩展欧几里得算法
s = 0; t = 1; old_s = 1; old_t = 0; r = run_mod(poly1, N);
old_r = run_mod(poly2, N);
while (length(old_r) > 0)
quo = run_div(r, old_r);
tmp_quo = cellfun(@(x) N-x, num2cell(quo));
tmp1 = run_poly_prod(tmp_quo, s);
tmp2 = run_poly_prod(tmp_quo, t);
tmp1 = run_mod(tmp1, N);
tmp2 = run_mod(tmp2, N);
tmp3 = run_poly_sum(old_s, tmp1);
tmp4 = run_poly_sum(old_t, tmp2);
old_s = s; s = tmp3;
old_t = t; t = tmp4;
tmp5 = old_r; old_r = r;
tmp6 = run_poly_prod(quo, old_r); tmp6 = run_mod(tmp6, N);
r = run_poly_sum(tmp5, -tmp6);
end
res = {old_r, old_s, old_t};
end
function res = run_poly_sum(poly1, poly2)
% 对两个多项式进行求和
[len1, len2] = deal(length(poly1), length(poly2));
if (len1 > len2)
padding = zeros(1, len1 - len2);
poly2 = [poly2 padding];
elseif (len1 < len2)
padding = zeros(1, len2 - len1);
poly1 = [poly1 padding];
end
res = poly1 + poly2;
end
function res = run_poly_prod(poly1, poly2)
% 对两个多项式进行乘法
len1 = length(poly1); len2 = length(poly2);
res = zeros(1, len1+len2-1);
for i=1:len1
for j=1:len2
res(i+j-1) = res(i+j-1) + poly1(i) * poly2(j);
end
end
end
function [res1, res2] = run_div(poly1, poly2)
% 对两个多项式进行除法
len1 = length(poly1); len2 = length(poly2);
if (len1 < len2)
res1 = [];
res2 = poly1;
else
quo = zeros(1, len1-len2+1);
while (len1 >= len2)
padding = zeros(1, len1-len2);
shifted_poly2 = [poly2 padding];
quot_elem = run_mod({poly1(len1)}, 2) / run_mod({shifted_poly2(len1)}, 2);
new_quo = zeros(1, len1-len2+1);
new_quo(len1-len2+1) = quot_elem{1};
quo = run_poly_sum(quo, new_quo);
multiplied_poly2 = run_poly_prod(new_quo, poly2);
poly1 = run_poly_sum(poly1, -multiplied_poly2);
len1 = length(poly1);
end
res1 = quo;
res2 = poly1;
end
end
function res = poly_rand(range, nonzero)
% 生成值在range范围内,非零项数目为nonzero的多项式
nonzero_indexes = randperm(range(2)-range(1)+1, nonzero) + range(1) - 1;
res = zeros(1, range(2)-range(1)+1);
res(nonzero_indexes) = randi([-1 1]); % 可以再指定范围[-p, p]修改生成的数值
end
```
请记住,这仅是一个基础的实现。在生产代码中,需要进行更多的优化和安全考虑。
相关推荐













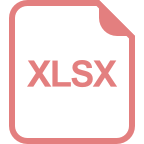
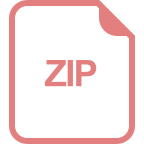