设计三星公司的设备管理系统。三星公司现有以下设备:普通电视机、DVD、带DVD的电视机,带DVD的电视机的售价为普通电视机和DVD单价之和的80%。请编制-程序要求对所有的库存设备都能实现按照品名进行显示、查找、增加和删除及保存的功能。c语言 需要给出fopen文件的内容
时间: 2024-02-27 20:57:26 浏览: 28
以下是一个简单的设备管理系统的C语言实现,包括了设备的添加、删除、查找和保存功能。为了方便,我们将设备信息存储在一个结构体数组中,每个元素表示一个设备,结构体中包括设备名称、类型和价格等信息。在程序中,我们使用文件来保存设备信息,使用fopen函数打开文件,使用fwrite和fread函数将设备信息写入和读取出来。
```c
#include <stdio.h>
#include <string.h>
#define MAX_DEVICES 100
// 定义设备类型枚举
enum DeviceType {
TV,
DVD,
TV_DVD
};
// 定义设备结构体
struct Device {
char name[20];
enum DeviceType type;
float price;
};
// 定义设备数组
struct Device devices[MAX_DEVICES];
// 定义设备数量
int deviceCount = 0;
// 显示设备信息
void showDevices() {
printf("设备列表:\n");
for (int i = 0; i < deviceCount; i++) {
printf("%s\t", devices[i].name);
switch(devices[i].type) {
case TV:
printf("普通电视机\t");
break;
case DVD:
printf("DVD\t");
break;
case TV_DVD:
printf("带DVD的电视机\t");
break;
default:
break;
}
printf("%.2f元\n", devices[i].price);
}
}
// 查找设备
void findDevice(char name[]) {
for (int i = 0; i < deviceCount; i++) {
if (strcmp(devices[i].name, name) == 0) {
printf("%s\t", devices[i].name);
switch(devices[i].type) {
case TV:
printf("普通电视机\t");
break;
case DVD:
printf("DVD\t");
break;
case TV_DVD:
printf("带DVD的电视机\t");
break;
default:
break;
}
printf("%.2f元\n", devices[i].price);
return;
}
}
printf("没有找到设备:%s\n", name);
}
// 添加设备
void addDevice(char name[], enum DeviceType type, float price) {
if (deviceCount == MAX_DEVICES) {
printf("设备数量已达上限,无法添加设备!\n");
return;
}
strcpy(devices[deviceCount].name, name);
devices[deviceCount].type = type;
if (type == TV_DVD) {
devices[deviceCount].price = price * 0.8;
} else {
devices[deviceCount].price = price;
}
deviceCount++;
printf("设备添加成功!\n");
}
// 删除设备
void deleteDevice(char name[]) {
int index = -1;
for (int i = 0; i < deviceCount; i++) {
if (strcmp(devices[i].name, name) == 0) {
index = i;
break;
}
}
if (index == -1) {
printf("没有找到设备:%s\n", name);
return;
}
for (int i = index; i < deviceCount - 1; i++) {
devices[i] = devices[i + 1];
}
deviceCount--;
printf("设备删除成功!\n");
}
// 保存设备信息到文件
void saveDevices(char filename[]) {
FILE *fp = fopen(filename, "wb");
if (fp == NULL) {
printf("无法打开文件:%s\n", filename);
return;
}
fwrite(&deviceCount, sizeof(int), 1, fp);
fwrite(devices, sizeof(struct Device), deviceCount, fp);
fclose(fp);
printf("设备信息已保存到文件:%s\n", filename);
}
// 从文件中读取设备信息
void loadDevices(char filename[]) {
FILE *fp = fopen(filename, "rb");
if (fp == NULL) {
printf("无法打开文件:%s\n", filename);
return;
}
fread(&deviceCount, sizeof(int), 1, fp);
fread(devices, sizeof(struct Device), deviceCount, fp);
fclose(fp);
printf("从文件:%s 中读取到设备信息\n", filename);
}
int main() {
// 加载设备信息
loadDevices("devices.bin");
// 显示菜单
printf("欢迎使用三星设备管理系统!\n");
printf("1. 显示设备列表\n");
printf("2. 查找设备\n");
printf("3. 添加设备\n");
printf("4. 删除设备\n");
printf("5. 保存设备信息到文件\n");
printf("0. 退出\n");
int choice = -1;
while (choice != 0) {
printf("请选择操作:");
scanf("%d", &choice);
switch (choice) {
case 1:
showDevices();
break;
case 2:
printf("请输入要查找的设备名称:");
char name[20];
scanf("%s", name);
findDevice(name);
break;
case 3:
printf("请输入设备名称:");
char addName[20];
scanf("%s", addName);
printf("请选择设备类型(1.普通电视机 2.DVD 3.带DVD的电视机):");
int addType;
scanf("%d", &addType);
printf("请输入设备价格:");
float addPrice;
scanf("%f", &addPrice);
addDevice(addName, addType - 1, addPrice);
break;
case 4:
printf("请输入要删除的设备名称:");
char deleteName[20];
scanf("%s", deleteName);
deleteDevice(deleteName);
break;
case 5:
saveDevices("devices.bin");
break;
case 0:
break;
default:
printf("无效的操作!\n");
break;
}
}
return 0;
}
```
在上面的代码中,我们定义了一个设备类型的枚举和设备结构体,以及一个设备数组和设备数量变量。程序中的菜单提供了显示、查找、添加、删除和保存设备信息的功能。保存设备信息时,我们使用了二进制写入方式打开了一个名为devices.bin的文件,并使用fwrite函数将设备数量和设备数组写入文件中;加载设备信息时,我们使用了二进制读取方式打开了同样的文件,并使用fread函数将设备数量和设备数组读取出来。
相关推荐
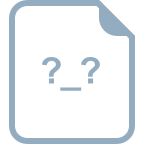
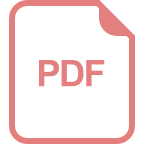














