本题要求编写复数类,该类至少包括加法、减法、乘法、除法的方法。还要编写ComplexDivException类,该类继承自Exception类,且至少包含两个数据成员,一个为错误代码,一个为错误信息。当复数类除数为0时,需要抛出该异常。编写测试类,测试以上两个类。
时间: 2024-02-12 13:07:05 浏览: 49
好的,我可以帮你解答这个问题。下面是复数类的代码实现:
```java
public class Complex {
private double real;
private double imag;
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public Complex add(Complex other) {
return new Complex(this.real + other.real, this.imag + other.imag);
}
public Complex subtract(Complex other) {
return new Complex(this.real - other.real, this.imag - other.imag);
}
public Complex multiply(Complex other) {
return new Complex(this.real * other.real - this.imag * other.imag, this.real * other.imag + this.imag * other.real);
}
public Complex divide(Complex other) throws ComplexDivException {
double denominator = other.real * other.real + other.imag * other.imag;
if (denominator == 0) {
throw new ComplexDivException("除数不能为0", 1);
} else {
return new Complex((this.real * other.real + this.imag * other.imag) / denominator,
(this.imag * other.real - this.real * other.imag) / denominator);
}
}
public String toString() {
return "(" + this.real + "," + this.imag + ")";
}
}
```
在上述代码中,我们定义了一个 Complex 类,并且实现了加、减、乘、除等基本运算。其中,除法操作可能会出现除数为0的情况,这时就需要抛出 ComplexDivException 异常。下面是 ComplexDivException 类的代码实现:
```java
public class ComplexDivException extends Exception {
private int errorCode;
private String errorMessage;
public ComplexDivException(String errorMessage, int errorCode) {
this.errorMessage = errorMessage;
this.errorCode = errorCode;
}
public int getErrorCode() {
return errorCode;
}
public String getErrorMessage() {
return errorMessage;
}
}
```
ComplexDivException 继承自 Exception 类,并且包含了一个错误代码和一个错误信息。当复数类的除数为0时,就会抛出该异常。
最后,我们可以编写一个测试类来测试上述两个类的功能:
```java
public class TestComplex {
public static void main(String[] args) {
Complex c1 = new Complex(1, 2);
Complex c2 = new Complex(3, 4);
Complex result = c1.add(c2);
System.out.println("c1 + c2 = " + result);
result = c1.subtract(c2);
System.out.println("c1 - c2 = " + result);
result = c1.multiply(c2);
System.out.println("c1 * c2 = " + result);
try {
result = c1.divide(new Complex(0, 0));
} catch (ComplexDivException e) {
System.out.println("除法出错:" + e.getErrorMessage() + ",错误代码为:" + e.getErrorCode());
}
try {
result = c1.divide(c2);
System.out.println("c1 / c2 = " + result);
} catch (ComplexDivException e) {
System.out.println("除法出错:" + e.getErrorMessage() + ",错误代码为:" + e.getErrorCode());
}
}
}
```
在测试类中,我们创建了两个复数对象 c1 和 c2,并且对它们进行了加、减、乘、除等操作。其中,在除法操作时,我们分别测试了除数为0和正常除法的情况。如果出现除数为0的情况,就会抛出 ComplexDivException 异常,并且在控制台输出错误信息和错误代码。
希望这个例子能够帮助你理解如何编写复数类和自定义异常类。
阅读全文
相关推荐


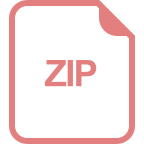
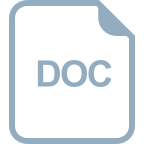
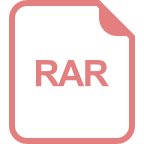
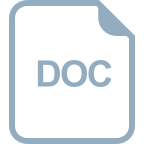
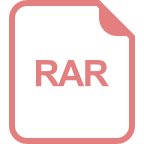
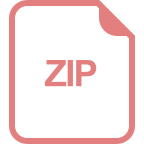
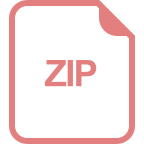
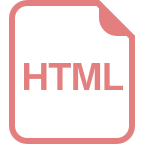
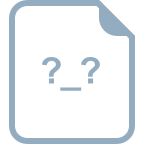