编写复数类,该类至少包括计算复数的加法、减法、乘法、除法的方法。 编写complexdivexception类,该类继承exception类,且至少包含两个数据成员,一个为错误代码,一个为错误信息。当复数类除数为0时要抛出该异常。 编写测试类,测试以上两个类。
时间: 2023-05-31 22:18:10 浏览: 254
### 回答1:
本题要求编写复数类,该类至少包括加法、减法、乘法、除法的方法。还要编写ComplexDivException类,该类继承自Exception类,且至少包含两个数据成员,一个为错误代码,一个为错误信息。当复数类除数为0时,需要抛出该异常。编写测试类,测试以上两个类。
### 回答2:
复数是由实数和虚数构成的数,其中虚数的系数i定义为i^2=-1。为了方便计算,我们需要创建一个复数类,能够实现对复数的加法、减法、乘法和除法运算。
要创建一个复数类,我们需要创建一个包含两个成员变量的类,分别表示实数部分和虚数部分。同时,我们需要实现四个方法:add,subtract,multiply和divide,分别表示加法、减法、乘法和除法运算。
在复数类的divide方法中,如果除数为0,我们需要抛出一个异常,告知用户不能除以0。为了实现这个功能,我们需要创建一个名为complexdivexception的类,它继承自标准异常类exception。此外,该类还需要包含两个数据成员,其中一个表示错误代码,另一个则表示错误信息。
在测试类中,我们需要创建两个复数对象,并测试它们的加法、减法、乘法和除法运算。如果有除数为0的情况发生,我们需要捕获complexdivexception异常,并输出相应的错误信息。
### 回答3:
复数是由实部和虚部组成的,可以用a+bi表示。因此,在编写复数类时,成员变量应该包括实部和虚部,同时需要实现加法、减法、乘法、除法等运算方法。
下面是复数类的代码示例:
```
public class Complex {
private double real;
private double imaginary;
public Complex(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
public double getReal() {
return real;
}
public double getImaginary() {
return imaginary;
}
// 加法
public Complex add(Complex other) {
double realPart = real + other.getReal();
double imaginaryPart = imaginary + other.getImaginary();
return new Complex(realPart, imaginaryPart);
}
// 减法
public Complex subtract(Complex other) {
double realPart = real - other.getReal();
double imaginaryPart = imaginary - other.getImaginary();
return new Complex(realPart, imaginaryPart);
}
// 乘法
public Complex multiply(Complex other) {
double realPart = real * other.getReal() - imaginary * other.getImaginary();
double imaginaryPart = real * other.getImaginary() + imaginary * other.getReal();
return new Complex(realPart, imaginaryPart);
}
// 除法(需要判断除数是否为0)
public Complex divide(Complex other) throws ComplexDivException {
double denominator = other.getReal() * other.getReal() + other.getImaginary() * other.getImaginary();
if (denominator == 0) {
throw new ComplexDivException("除数不能为0", 1001);
}
double realPart = (real * other.getReal() + imaginary * other.getImaginary()) / denominator;
double imaginaryPart = (imaginary * other.getReal() - real * other.getImaginary()) / denominator;
return new Complex(realPart, imaginaryPart);
}
@Override
public String toString() {
return real + " + " + imaginary + "i";
}
}
```
在上面的代码中,除法方法中如果分母为0,则抛出ComplexDivException异常。下面是ComplexDivException的代码示例:
```
public class ComplexDivException extends Exception {
private int errorCode;
private String errorMessage;
public ComplexDivException(String errorMessage, int errorCode) {
this.errorMessage = errorMessage;
this.errorCode = errorCode;
}
public int getErrorCode() {
return errorCode;
}
public String getErrorMessage() {
return errorMessage;
}
@Override
public String toString() {
return "ComplexDivException{" +
"errorCode=" + errorCode +
", errorMessage='" + errorMessage + '\'' +
'}';
}
}
```
在测试类中,可以编写代码对复数类和ComplexDivException类进行测试。下面是测试类的代码示例:
```
public class ComplexTest {
public static void main(String[] args) {
Complex c1 = new Complex(3, 4);
Complex c2 = new Complex(1, 2);
System.out.println("c1 = " + c1);
System.out.println("c2 = " + c2);
System.out.println("c1 + c2 = " + c1.add(c2));
System.out.println("c1 - c2 = " + c1.subtract(c2));
System.out.println("c1 * c2 = " + c1.multiply(c2));
try {
System.out.println("c1 / c2 = " + c1.divide(c2));
} catch (ComplexDivException e) {
System.out.println("除数为0,错误代码:" + e.getErrorCode() + ", 错误信息:" + e.getErrorMessage());
}
Complex c3 = new Complex(5, 0);
Complex c4 = new Complex(0, 2);
System.out.println("c3 = " + c3);
System.out.println("c4 = " + c4);
System.out.println("c3 + c4 = " + c3.add(c4));
System.out.println("c3 - c4 = " + c3.subtract(c4));
System.out.println("c3 * c4 = " + c3.multiply(c4));
try {
System.out.println("c3 / c4 = " + c3.divide(c4));
} catch (ComplexDivException e) {
System.out.println("除数为0,错误代码:" + e.getErrorCode() + ", 错误信息:" + e.getErrorMessage());
}
}
}
```
在上面的测试类中,创建了多个复数对象来进行加减乘除等运算,其中也包括了除数为0的情况,测试了ComplexDivException的异常处理。运行测试类后,可以看到输出了复数的运算结果以及除数为0时的异常信息。
阅读全文
相关推荐



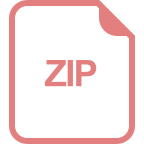
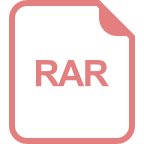
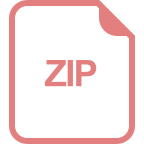
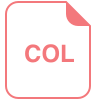







