c++编写程序利用重载运算符实现复数类对象的算术四则运算
时间: 2023-08-02 13:07:49 浏览: 152
好的,我可以帮你解答这个问题。以下是一个简单的复数类的代码示例,其中包括了算术四则运算符的重载实现。
```c++
#include <iostream>
using namespace std;
class Complex {
public:
Complex(double real = 0.0, double imag = 0.0) : re(real), im(imag) {}
Complex operator+(const Complex& c) const {
return Complex(re + c.re, im + c.im);
}
Complex operator-(const Complex& c) const {
return Complex(re - c.re, im - c.im);
}
Complex operator*(const Complex& c) const {
return Complex(re * c.re - im * c.im, re * c.im + im * c.re);
}
Complex operator/(const Complex& c) const {
double denominator = c.re * c.re + c.im * c.im;
return Complex((re * c.re + im * c.im) / denominator,
(im * c.re - re * c.im) / denominator);
}
friend ostream& operator<<(ostream& os, const Complex& c) {
os << "(" << c.re << ", " << c.im << "i)";
return os;
}
private:
double re, im;
};
int main() {
Complex a(1.0, 2.0);
Complex b(3.0, 4.0);
Complex c = a + b;
Complex d = a - b;
Complex e = a * b;
Complex f = a / b;
cout << "a + b = " << c << endl;
cout << "a - b = " << d << endl;
cout << "a * b = " << e << endl;
cout << "a / b = " << f << endl;
return 0;
}
```
在这个示例中,我们定义了一个复数类 `Complex`,其中包含实部和虚部两个私有数据成员。我们通过重载运算符 `+`、`-`、`*` 和 `/` 来实现了相应的算术四则运算。此外,我们还定义了一个友元函数 `operator<<`,用于输出复数类对象的值。
在 `main` 函数中,我们创建了两个 `Complex` 类对象 `a` 和 `b`,并对其进行了加、减、乘、除四种运算操作。最后,我们通过 `cout` 输出了运算结果。
希望这个示例能够帮助你了解如何利用重载运算符实现复数类对象的算术四则运算。
阅读全文
相关推荐





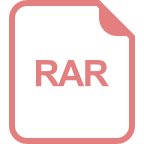












