c++用运算符重载设计复数类,实现复数的+、-、*、/运算。
时间: 2024-12-18 18:20:11 浏览: 6
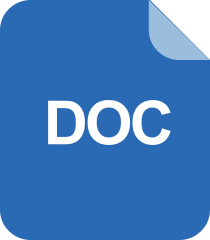
实验五、运算符重载 复数+-*/ 大数+-*/
在 C++ 中,可以利用运算符重载(operator overloading)来设计复数类(Complex),使得我们可以像操作普通数值一样直接对复数进行加减乘除等操作。首先,我们创建一个名为 Complex 的结构体或类,包含两个成员变量代表实部(real)和虚部(imaginary)。然后,我们定义运算符重载函数,分别对应四种基本算术运算:
```cpp
class Complex {
public:
double real, imag;
// 构造函数
Complex(double r = 0.0, double i = 0.0) : real(r), imag(i) {}
// 运算符重载 - 加法
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
// 运算符重载 - 减法
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
// 运算符重载 - 乘法
Complex operator*(const Complex& other) const {
double newReal = real * other.real - imag * other.imag;
double newImag = real * other.imag + imag * other.real;
return Complex(newReal, newImag);
}
// 运算符重载 - 除法
Complex operator/(const Complex& other) const {
double denominator = other.real * other.real + other.imag * other.imag;
if (denominator == 0)
throw std::runtime_error("Division by zero is not allowed for complex numbers.");
double newReal = (real * other.real + imag * other.imag) / denominator;
double newImag = (imag * other.real - real * other.imag) / denominator;
return Complex(newReal, newImag);
}
};
// 使用示例
int main() {
Complex a(1, 2);
Complex b(3, 4);
Complex sum = a + b; // a + b 的结果
Complex diff = a - b; // a - b 的结果
Complex product = a * b; // a * b 的结果
Complex quotient = a / b; // a / b 的结果
return 0;
}
```
阅读全文
相关推荐
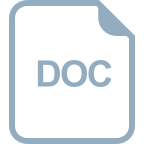
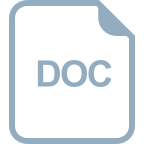





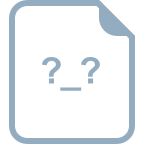






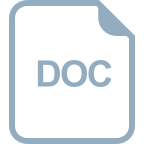
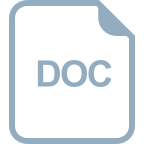
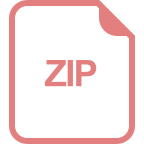
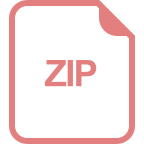