1.定义一个复数类Complex,重载前置自增“++”运算符及后置自增“++”运算符,使之能用于复数的自增运算。 注:复数的自增是让复数的实部和虚部同时增加1.主函数示例如下: #include <iostream> using namespace std; /*********在begin和end之间填入代码,实现complex类的定义,重载前置++运算符和重载后置++运算符及display()函数********/ /***********begin*************/ void Complex::display() { if(real==0) { if(imag>0) cout<<imag<<"i"; else if(imag==0) cout<<real; else cout<<imag<<"i"; } else { if(imag>0) cout<<real<<"+"<<imag<<"i"; else if(imag==0) cout<<real; else cout<<real<<imag<<"i"; } } /***********end************/ int main() { Complex c1,c2; cin>>c1.real>>c1.imag; c1.display(); cout<<endl; ++c1; c2=c1++; c2.display(); cout<<endl; c1.display(); } 2.已知矩阵为2行3列,重载流插入运算符“<<”和流提取运算符">>",使之能完成矩阵的输入和输出。 主函数示例如下: #include <iostream> using namespace std; //请在begin和end间完成Matrix类的编写,重载>>及<<运算符,建议重载为友元函数 /*********begin**********/ /*********end*********/ int main() { Matrix m1; cin>>m1; cout<<"output matrix"<<endl; cout<<m1; }
时间: 2023-07-06 08:37:22 浏览: 172
下面是两个问题的代码实现:
1. 定义一个复数类Complex,重载前置自增“++”运算符及后置自增“++”运算符,使之能用于复数的自增运算。
```c++
#include <iostream>
using namespace std;
class Complex {
private:
double real, imag;
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// 前置自增
Complex& operator++() {
real++;
imag++;
return *this;
}
// 后置自增
Complex operator++(int) {
Complex temp(*this);
real++;
imag++;
return temp;
}
void display() {
if (real == 0) {
if (imag > 0)
cout << imag << "i";
else if (imag == 0)
cout << real;
else
cout << imag << "i";
}
else {
if (imag > 0)
cout << real << "+" << imag << "i";
else if (imag == 0)
cout << real;
else
cout << real << imag << "i";
}
}
};
int main() {
Complex c1, c2;
cin >> c1.real >> c1.imag;
c1.display();
cout << endl;
++c1;
c2 = c1++;
c2.display();
cout << endl;
c1.display();
return 0;
}
```
在上面的代码中,我们重载了前置自增和后置自增运算符,并定义了一个display()函数,用于输出复数对象的值。在主函数中,我们创建了两个复数对象,对它们进行了自增运算,并输出了结果。
2. 已知矩阵为2行3列,重载流插入运算符“<<”和流提取运算符“>>”,使之能完成矩阵的输入和输出。
```c++
#include <iostream>
using namespace std;
class Matrix {
private:
int data[2][3];
public:
friend istream& operator>>(istream& is, Matrix& m) {
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
is >> m.data[i][j];
}
}
return is;
}
friend ostream& operator<<(ostream& os, const Matrix& m) {
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
os << m.data[i][j] << " ";
}
os << endl;
}
return os;
}
};
int main() {
Matrix m1;
cin >> m1;
cout << "output matrix" << endl;
cout << m1;
return 0;
}
```
在上面的代码中,我们定义了一个Matrix类,其中包含了一个2行3列的矩阵。我们重载了流插入运算符“>>”和流提取运算符“<<”,并实现了矩阵的输入和输出。在主函数中,我们创建了一个Matrix对象,对它进行了输入,并输出了结果。
阅读全文
相关推荐
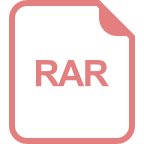
















