写一个C++程序要求包括(1)类的定义,构造函数,成员函数 (2).两个复数运算的运算符重载.重载 +、-、*、/、++、--、<<、>>等运算符 (3) double型数可以转换成复数
时间: 2023-08-25 10:04:41 浏览: 57
好的,以下是一个完整的 C++ 程序,满足您的要求:
```cpp
#include <iostream>
using namespace std;
class Complex {
private:
double real; // 实部
double imag; // 虚部
public:
Complex() { // 默认构造函数
real = 0;
imag = 0;
}
Complex(double r, double i) { // 带参数构造函数
real = r;
imag = i;
}
Complex(const Complex& c) { // 拷贝构造函数
real = c.real;
imag = c.imag;
}
~Complex() { // 析构函数
// do nothing
}
void setReal(double r) { // 设置实部
real = r;
}
void setImag(double i) { // 设置虚部
imag = i;
}
double getReal() const { // 获取实部
return real;
}
double getImag() const { // 获取虚部
return imag;
}
Complex operator+(const Complex& c) const { // 运算符重载 +
return Complex(real + c.real, imag + c.imag);
}
Complex operator-(const Complex& c) const { // 运算符重载 -
return Complex(real - c.real, imag - c.imag);
}
Complex operator*(const Complex& c) const { // 运算符重载 *
return Complex(real * c.real - imag * c.imag, real * c.imag + imag * c.real);
}
Complex operator/(const Complex& c) const { // 运算符重载 /
double denominator = c.real * c.real + c.imag * c.imag;
return Complex((real * c.real + imag * c.imag) / denominator,
(imag * c.real - real * c.imag) / denominator);
}
Complex operator++() { // 运算符重载前置 ++
real++;
imag++;
return *this;
}
Complex operator++(int) { // 运算符重载后置 ++
Complex tmp(*this);
real++;
imag++;
return tmp;
}
Complex operator--() { // 运算符重载前置 --
real--;
imag--;
return *this;
}
Complex operator--(int) { // 运算符重载后置 --
Complex tmp(*this);
real--;
imag--;
return tmp;
}
friend istream& operator>>(istream& is, Complex& c) { // 运算符重载 >>
is >> c.real >> c.imag;
return is;
}
friend ostream& operator<<(ostream& os, const Complex& c) { // 运算符重载 <<
os << c.real << " + " << c.imag << "i";
return os;
}
explicit operator double() const { // 类型转换函数
return real;
}
};
int main() {
Complex c1(1.0, 2.0);
Complex c2(3.0, 4.0);
// 运算符重载
Complex c3 = c1 + c2;
Complex c4 = c1 - c2;
Complex c5 = c1 * c2;
Complex c6 = c1 / c2;
Complex c7 = ++c1;
Complex c8 = c2++;
Complex c9 = --c1;
Complex c10 = c2--;
cout << "c1 = " << c1 << endl;
cout << "c2 = " << c2 << endl;
cout << "c1 + c2 = " << c3 << endl;
cout << "c1 - c2 = " << c4 << endl;
cout << "c1 * c2 = " << c5 << endl;
cout << "c1 / c2 = " << c6 << endl;
cout << "++c1 = " << c7 << endl;
cout << "c2++ = " << c8 << endl;
cout << "--c1 = " << c9 << endl;
cout << "c2-- = " << c10 << endl;
// 类型转换
double d = (double)c1;
cout << "double(c1) = " << d << endl;
return 0;
}
```
程序中定义了一个 `Complex` 类,实现了复数的加、减、乘、除、前置/后置自增/自减、输入输出运算符重载以及类型转换。在 `main` 函数中进行了测试。
相关推荐
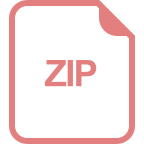
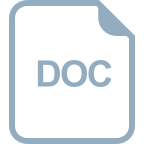
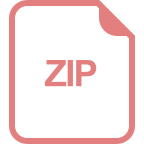














