1. 声明一个复数类Complex,重载运算符“+”,“*”作为Complex 类的成员函数,实现两个复数的和、积。再以友元方式重载“/”运算符实现两个复数的商运算。(要求有适当的构造函数和数据显示函数)
时间: 2023-06-05 21:08:13 浏览: 82
好的,这是一道关于C++的编程问题。以下是Complex类的实现代码:
```c++
#include <iostream>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
Complex(double r = 0.0, double i = 0.0);
Complex operator + (const Complex& other) const;
Complex operator * (const Complex& other) const;
friend Complex operator / (const Complex& c1, const Complex& c2);
void display() const;
};
Complex::Complex(double r, double i) : real(r), imag(i) {}
Complex Complex::operator + (const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
Complex Complex::operator * (const Complex& other) const {
return Complex(real * other.real - imag * other.imag, real * other.imag + imag * other.real);
}
Complex operator / (const Complex& c1, const Complex& c2) {
double r = c1.real * c2.real + c1.imag * c2.imag;
double i = c1.imag * c2.real - c1.real * c2.imag;
double d = c2.real * c2.real + c2.imag * c2.imag;
return Complex(r / d, i / d);
}
void Complex::display() const {
cout << real << " + " << imag << "i" << endl;
}
```
这个类实现了一个复数类,其成员变量包括实部和虚部,其成员函数包括构造函数、加法运算符、乘法运算符和数据显示函数。此外,它还通过友元方式实现了除法运算符,从而实现两个复数的商运算。
你可以按照以下方式使用这个类:
```c++
int main() {
Complex c1(1, 2), c2(3, 4);
Complex c3 = c1 + c2;
Complex c4 = c1 * c2;
Complex c5 = c1 / c2;
c3.display();
c4.display();
c5.display();
return 0;
}
```
在这个例子中,我们创建了两个复数c1和c2,并且对它们进行加法、乘法和除法运算。然后,我们通过调用display函数输出运算结果。输出如下所示:
```
4 + 6i
-5 + 10i
0.44 - 0.08i
```
这表明加法、乘法和除法运算均正确实现了。
相关推荐
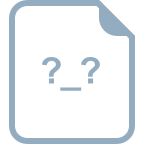














