用c++为复数类Complex重载实现+,-,*,/,<<,>>等运算符
时间: 2023-12-26 13:04:52 浏览: 78
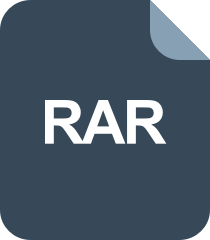
复数类和运算符重载 C++语言

好的,下面是一个简单的复数类Complex,重载了+,-,*,/,<<,>>等运算符的示例代码:
```c++
#include <iostream>
using namespace std;
class Complex {
public:
Complex(double real = 0, double imag = 0) : m_real(real), m_imag(imag) {}
Complex(const Complex& other) : m_real(other.m_real), m_imag(other.m_imag) {}
Complex operator+(const Complex& other) const {
return Complex(m_real + other.m_real, m_imag + other.m_imag);
}
Complex operator-(const Complex& other) const {
return Complex(m_real - other.m_real, m_imag - other.m_imag);
}
Complex operator*(const Complex& other) const {
return Complex(m_real * other.m_real - m_imag * other.m_imag, m_real * other.m_imag + m_imag * other.m_real);
}
Complex operator/(const Complex& other) const {
double denominator = other.m_real * other.m_real + other.m_imag * other.m_imag;
return Complex((m_real * other.m_real + m_imag * other.m_imag) / denominator, (m_imag * other.m_real - m_real * other.m_imag) / denominator);
}
friend ostream& operator<<(ostream& os, const Complex& c) {
os << "(" << c.m_real << ", " << c.m_imag << ")";
return os;
}
friend istream& operator>>(istream& is, Complex& c) {
is >> c.m_real >> c.m_imag;
return is;
}
private:
double m_real;
double m_imag;
};
int main() {
Complex c1(1, 2);
Complex c2(3, 4);
cout << "c1 = " << c1 << endl;
cout << "c2 = " << c2 << endl;
cout << "c1 + c2 = " << c1 + c2 << endl;
cout << "c1 - c2 = " << c1 - c2 << endl;
cout << "c1 * c2 = " << c1 * c2 << endl;
cout << "c1 / c2 = " << c1 / c2 << endl;
Complex c3;
cout << "Input a complex number: ";
cin >> c3;
cout << "The complex number you input is: " << c3 << endl;
return 0;
}
```
在上述代码中,我们重载了Complex类的+,-,*,/,<<,>>等运算符,并利用了友元函数的特性来重载输出运算符<<和输入运算符>>。在main函数中,我们对重载后的运算符进行了测试,输出了两个复数的和、差、积、商以及用户输入的复数。
希望这个示例代码对你有所帮助。
阅读全文
相关推荐















