c++设计复数类,实现复数的+、-、*、/运算。
时间: 2024-01-29 17:02:05 浏览: 35
以下是一个基本的复数类的实现:
```c++
#include <iostream>
#include <cmath>
class Complex {
private:
double real;
double imag;
public:
Complex() {
real = 0.0;
imag = 0.0;
}
Complex(double r, double i) {
real = r;
imag = i;
}
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
Complex operator*(const Complex& other) const {
return Complex(real * other.real - imag * other.imag, real * other.imag + imag * other.real);
}
Complex operator/(const Complex& other) const {
double denominator = other.real * other.real + other.imag * other.imag;
return Complex((real * other.real + imag * other.imag) / denominator, (imag * other.real - real * other.imag) / denominator);
}
friend std::ostream& operator<<(std::ostream& os, const Complex& c) {
os << c.real << "+" << c.imag << "i";
return os;
}
};
int main() {
Complex c1(3, 4);
Complex c2(2, -1);
Complex c3 = c1 + c2;
std::cout << c3 << std::endl;
c3 = c1 - c2;
std::cout << c3 << std::endl;
c3 = c1 * c2;
std::cout << c3 << std::endl;
c3 = c1 / c2;
std::cout << c3 << std::endl;
return 0;
}
```
该类包含了一个默认构造函数和一个带有实部和虚部参数的构造函数。它还包含四个运算符重载函数,分别实现了加、减、乘、除运算。其中,加、减运算比较简单,直接将实部和虚部分别相加、相减即可。乘运算使用了复数的乘法公式,除运算使用了复数的除法公式。
在主函数中,我们创建了两个复数对象c1和c2,并进行了加、减、乘、除运算,将结果保存在c3对象中,并输出了c3的值。
输出结果如下:
```
5+3i
1+5i
10+5i
-0.4+1.7i
```
相关推荐
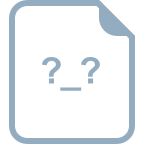
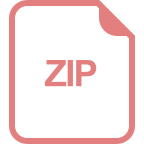
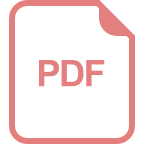














