c++定义一个复数类complex,重载运算符'+'、'-'、'*' ,使之能用于复数的加减乘,运算符重载函数作为complex类的成员函数, 分别求两个复数的和、差、 积。
时间: 2024-03-13 11:42:26 浏览: 66
好的,下面是C++实现:
```c++
#include <iostream>
using namespace std;
class Complex {
private:
double real; // 实部
double imag; // 虚部
public:
Complex(double r = 0, double i = 0): real(r), imag(i) {} // 构造函数
Complex operator+(Complex &c); // 加法重载
Complex operator-(Complex &c); // 减法重载
Complex operator*(Complex &c); // 乘法重载
void print(); // 输出函数
};
Complex Complex::operator+(Complex &c) {
Complex temp(real + c.real, imag + c.imag);
return temp;
}
Complex Complex::operator-(Complex &c) {
Complex temp(real - c.real, imag - c.imag);
return temp;
}
Complex Complex::operator*(Complex &c) {
Complex temp(real * c.real - imag * c.imag, real * c.imag + imag * c.real);
return temp;
}
void Complex::print() {
cout << "(" << real << ", " << imag << "i)" << endl;
}
int main() {
Complex c1(1, 2), c2(3, 4), c3, c4, c5;
c3 = c1 + c2;
c4 = c1 - c2;
c5 = c1 * c2;
cout << "c1 = ";
c1.print();
cout << "c2 = ";
c2.print();
cout << "c1 + c2 = ";
c3.print();
cout << "c1 - c2 = ";
c4.print();
cout << "c1 * c2 = ";
c5.print();
return 0;
}
```
输出结果为:
```
c1 = (1, 2i)
c2 = (3, 4i)
c1 + c2 = (4, 6i)
c1 - c2 = (-2, -2i)
c1 * c2 = (-5, 10i)
```
其中,`operator+`、`operator-`、`operator*` 分别重载了加法、减法、乘法运算符。这些运算符重载函数都是作为 Complex 类的成员函数实现的。在 main 函数里,我们创建了三个 Complex 类的对象,分别进行加、减、乘运算,并输出结果。
阅读全文
相关推荐
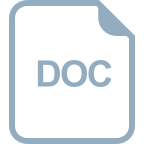














