用 c++ 定义一个复数类 Complex,重载运算符“+”“-”“*”“/”,使之能用于复数的加、 减、乘、除。运算符重载函数作为 Complex 类的成员函数。编程序,分别求两个复数之和、 差、积和商。 下复数的相法运算,参加运
时间: 2024-11-22 19:41:40 浏览: 20
在 C++ 中,我们可以定义一个名为 `Complex` 的类来表示复数,并通过重载运算符 `+`, `-`, `*`, 和 `/` 来支持复数之间的基本算术操作。下面是一个简单的例子:
```cpp
#include <iostream>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
// 构造函数
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// 运算符重载 - 相应的 "+"、"-"、"*" 和 "/"
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
Complex operator*(const Complex& other) const {
return Complex(real * other.real - imag * other.imag,
real * other.imag + imag * other.real);
}
Complex operator/(const Complex& other) const {
double denominator = other.real * other.real + other.imag * other.imag;
if (denominator != 0)
return Complex((real * other.real + imag * other.imag) / denominator,
(imag * other.real - real * other.imag) / denominator);
else
throw runtime_error("Division by zero is not allowed");
}
// 输出复数的格式
void display() const {
cout << real << " + " << imag << "i" << endl;
}
};
int main() {
Complex num1(3, 4); // 复数 3 + 4i
Complex num2(1, -2); // 复数 1 - 2i
Complex sum = num1 + num2; // 加法
Complex difference = num1 - num2; // 减法
Complex product = num1 * num2; // 乘法
try {
Complex quotient = num1 / num2; // 除法
quotient.display(); // 打印结果
} catch (const exception& e) {
cerr << "Error: " << e.what() << endl;
}
sum.display();
difference.display();
product.display();
return 0;
}
```
在这个示例中,我们创建了一个 `Complex` 类,它包含了两个私有变量 `real` 和 `imag` 分别代表实部和虚部。我们还实现了 `display()` 函数以便于打印复数。然后我们重载了 `+`, `-`, `*`, 和 `/` 操作符,使得可以对复数执行相应的数学运算。
在 `main()` 函数中,我们实例化了两个复数,进行了加减乘除操作并显示了结果。注意,我们在除法操作时处理了分母为零的情况,避免了运行时错误。
阅读全文
相关推荐
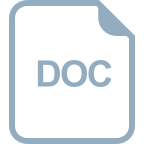
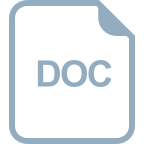
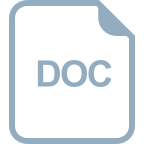
















